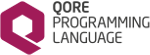 |
Qore Programming Language
0.9.16
|
32 #ifndef _QORE_ABSTRACTQORENODE_H
34 #define _QORE_ABSTRACTQORENODE_H
37 #include <qore/QoreReferenceCounter.h>
44 #define FMT_YAML_SHORT -2
64 DLLEXPORT
AbstractQoreNode(
qore_type_t t,
bool n_value,
bool n_needs_eval,
bool n_there_can_be_only_one =
false,
bool n_custom_reference_handlers =
false);
180 DLLEXPORT
virtual const char*
getTypeName()
const = 0;
238 DLLEXPORT
void ref()
const;
251 DLLLOCAL
virtual void parseInit(
QoreValue& val, LocalVar* oflag,
int pflag,
int& lvids,
const QoreTypeInfo*& typeInfo);
308 DLLEXPORT
virtual void customRef()
const;
362 DLLEXPORT
void deref();
389 DLLLOCAL
void *
operator new(size_t) =
delete;
DLLLOCAL SimpleQoreNode(qore_type_t t, bool n_value, bool n_needs_eval, bool n_there_can_be_only_one=false)
constructor takes the type and value arguments
Definition: AbstractQoreNode.h:352
DLLLOCAL bool isReferenceCounted() const
returns true if the object is reference-counted
Definition: AbstractQoreNode.h:241
DLLLOCAL bool needs_eval() const
returns true if the object needs evaluation to return a value, false if not
Definition: AbstractQoreNode.h:142
virtual DLLLOCAL bool getAsBoolImpl() const
default implementation, returns false
Definition: AbstractQoreNode.h:260
virtual DLLLOCAL double getAsFloatImpl() const
default implementation, returns 0.0
Definition: AbstractQoreNode.h:278
bool value
this is true for values, if false then either the type needs evaluation to produce a value or is a pa...
Definition: AbstractQoreNode.h:324
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
virtual const DLLEXPORT char * getTypeName() const =0
returns the type name as a c string
DLLEXPORT AbstractQoreNode * refSelf() const
returns "this" with an incremented reference count
virtual DLLEXPORT class DateTime * getDateTimeRepresentation(bool &del) const
returns the DateTime representation of this type (default implementation: returns ZeroDate,...
bool there_can_be_only_one
if this is set to true, then reference counting is turned off for objects of this class
Definition: AbstractQoreNode.h:330
virtual DLLEXPORT void customDeref(ExceptionSink *xsink)
DLLEXPORT bool getAsBool() const
returns the boolean value of the object
virtual DLLEXPORT bool derefImpl(ExceptionSink *xsink)
decrements the reference count
DLLLOCAL UniqueValueQoreNode(qore_type_t t)
constructor takes the type argument
Definition: AbstractQoreNode.h:392
bool custom_reference_handlers
set to one for objects that need custom reference handlers
Definition: AbstractQoreNode.h:333
DLLLOCAL qore_type_t getType() const
returns the data type
Definition: AbstractQoreNode.h:172
virtual DLLEXPORT bool is_equal_hard(const AbstractQoreNode *v, ExceptionSink *xsink) const =0
tests for equality ("deep compare" including all contained values for container types) without type c...
virtual DLLEXPORT ~AbstractQoreNode()
default destructor does nothing
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
virtual DLLEXPORT AbstractQoreNode * realCopy() const
returns itself; objects of this type are not reference-counted and only deleted manually (by static d...
virtual DLLLOCAL int64 getAsBigIntImpl() const
default implementation, returns 0
Definition: AbstractQoreNode.h:272
DLLLOCAL bool is_value() const
returns true if the node represents a value
Definition: AbstractQoreNode.h:220
Qore's string type supported by the QoreEncoding class.
Definition: QoreString.h:81
virtual DLLEXPORT AbstractQoreNode * realCopy() const =0
returns a copy of the object; the caller owns the reference count
virtual DLLEXPORT void customRef() const
special processing when the object's reference count transitions from 0-1
virtual DLLEXPORT int getAsString(QoreString &str, int foff, ExceptionSink *xsink) const =0
concatenate the verbose string representation of the value (including all contained values for contai...
bool needs_eval_flag
if this is true then the type can be evaluated
Definition: AbstractQoreNode.h:327
base class for simple value types
Definition: AbstractQoreNode.h:366
DLLLOCAL SimpleValueQoreNode(qore_type_t t, bool n_there_can_be_only_one=false)
creates the object by assigning the type code and setting the "value" flag, unsetting the "needs_eval...
Definition: AbstractQoreNode.h:369
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
DLLEXPORT QoreValue eval(ExceptionSink *xsink) const
evaluates the object and returns a value (or 0)
provides atomic reference counting to Qore objects
Definition: QoreReferenceCounter.h:44
this class is for value types that will exists only once in the Qore library, reference counting is d...
Definition: AbstractQoreNode.h:383
virtual DLLLOCAL int getAsIntImpl() const
default implementation, returns 0
Definition: AbstractQoreNode.h:266
virtual DLLEXPORT QoreValue evalImpl(bool &needs_deref, ExceptionSink *xsink) const =0
optionally evaluates the argument
DLLEXPORT void ref() const
increments the reference count
DLLLOCAL UniqueValueQoreNode & operator=(const UniqueValueQoreNode &)=delete
this function is not implemented
Holds absolute and relative date/time values in Qore with precision to the microsecond.
Definition: DateTime.h:93
DLLEXPORT double getAsFloat() const
returns the float value of the object
virtual DLLEXPORT QoreString * getStringRepresentation(bool &del) const
returns the value of the type converted to a string, default implementation: returns the empty string
DLLEXPORT void deref(ExceptionSink *xsink)
decrements the reference count and calls derefImpl() if there_can_be_only_one is false,...
DLLEXPORT int getAsInt() const
returns the integer value of the object
virtual DLLEXPORT bool is_equal_soft(const AbstractQoreNode *v, ExceptionSink *xsink) const =0
tests for equality ("deep compare" including all contained values for container types) with possible ...
qore_type_t type
the type of the object
Definition: AbstractQoreNode.h:321
DLLEXPORT AbstractQoreNode(qore_type_t t, bool n_value, bool n_needs_eval, bool n_there_can_be_only_one=false, bool n_custom_reference_handlers=false)
constructor takes the type
SimpleQoreNode & operator=(const SimpleQoreNode &)=delete
this function is not implemented
virtual DLLEXPORT QoreValue evalImpl(bool &needs_deref, ExceptionSink *xsink) const
should never be called for value types
The base class for all value and parse types in Qore expression trees.
Definition: AbstractQoreNode.h:54
int16_t qore_type_t
used to identify unique Qore data and parse types (descendents of AbstractQoreNode)
Definition: common.h:70
DLLEXPORT int64 getAsBigInt() const
returns the 64-bit integer value of the object
DLLLOCAL AbstractQoreNode & operator=(const AbstractQoreNode &)
this function is not implemented; it is here as a private function in order to prohibit it from being...
virtual DLLLOCAL void parseInit(QoreValue &val, LocalVar *oflag, int pflag, int &lvids, const QoreTypeInfo *&typeInfo)
for use by parse types to initialize them for execution during stage 1 parsing; not exported in the l...
The base class for all types in Qore expression trees that cannot throw an exception when deleted.
Definition: AbstractQoreNode.h:346