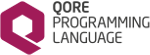 |
Qore Programming Language
0.9.16
|
34 #ifndef _QORE_DATASOURCE_H
36 #define _QORE_DATASOURCE_H
38 #include <qore/QoreThreadLock.h>
39 #include <qore/QoreQueue.h>
44 class DatasourceStatementHelper;
56 friend class QoreSQLStatement;
57 friend hashdecl qore_ds_private;
60 hashdecl qore_ds_private *priv;
202 template <
typename T>
208 template <
typename T>
210 return *getPrivateData<T>();
276 DLLEXPORT
void setAutoCommit(
bool ac);
368 DLLEXPORT
int close();
441 DLLEXPORT
bool isOpen()
const;
625 #endif // _QORE_DATASOURCE_H
DLLEXPORT Datasource * copy() const
returns a copy of this object with the same DBIDriver and pending connection values
const DLLEXPORT DBIDriver * getDriver() const
returns the DBIDriver pointer used for this object
const DLLEXPORT char * getOSEncoding() const
returns the OS (or Qore) character encoding name used for the last connection
const DLLEXPORT QoreEncoding * getQoreEncoding() const
returns the QoreEncoding pointer used for this connection
DLLEXPORT void setPendingUsername(const char *u)
sets the username to be used for the next connection
DLLEXPORT QoreStringNode * getPendingDBName() const
returns the pending database (or schema) name for the next connection
DLLEXPORT void setPendingHostName(const char *h)
sets the hostname to be used for the next connection
const DLLEXPORT std::string & getPasswordStr() const
returns the password used for the last connection
DLLEXPORT void reset(ExceptionSink *xsink)
closes and opens the connection
const DLLEXPORT char * getUsername() const
returns the username used for the last connection
DLLEXPORT void setDBEncoding(const char *name)
sets the database-specific character encoding name used for the current connection
const DLLEXPORT char * getPassword() const
returns the password used for the last connection
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
DLLEXPORT QoreStringNode * getPendingHostName() const
returns the pending host name for the next connection
DLLEXPORT QoreValue getOption(const char *opt, ExceptionSink *xsink)
Returns the current value for the given option.
DLLEXPORT bool isInTransaction() const
returns the transaction status flag
DLLEXPORT QoreValue selectRows(const QoreString *query_str, const QoreListNode *args, ExceptionSink *xsink)
executes SQL throught the "selectRows" function of the DBI driver and returns the result,...
This is the hash or associative list container type in Qore, dynamically allocated only,...
Definition: QoreHashNode.h:50
DLLEXPORT QoreHashNode * getCurrentOptionHash() const
returns the options currently set for this object
const DLLEXPORT std::string & getDBNameStr() const
returns the database (or schema) name used for the last connection
DLLEXPORT void setPendingPort(int port)
sets the port number to be used for the next connection
const DLLEXPORT std::string & getDBEncodingStr() const
returns the database-specific character encoding name used for the last connection
DLLEXPORT void setEventQueue(Queue *q, QoreValue arg, ExceptionSink *xsink)
sets an event queue for datasource events
DLLEXPORT bool isOpen() const
returns true if the connection is currently open
DLLEXPORT int getCapabilities() const
returns the capability mask of the current driver
const DLLEXPORT QoreHashNode * getConnectOptions() const
returns the valid options for this driver with descriptions and current values for the current dataso...
DLLEXPORT QoreStringNode * getPendingUsername() const
returns the pending username for the next connection
DLLEXPORT void setPendingDBName(const char *d)
sets the database (or schema) name to be used for the next connection
DLLEXPORT int commit(ExceptionSink *xsink)
commits the current transaction to the database
virtual DLLEXPORT ~Datasource()
the Datasource is closed if it's still open and the object is destroyed
DLLEXPORT int autoCommit(ExceptionSink *xsink)
called from subclasses when releasing the transaction lock
DLLEXPORT int getPort() const
returns the port number used for the last connection
DLLEXPORT QoreHashNode * getConfigHash() const
returns a hash representing the configuration of the current object
This is the list container type in Qore, dynamically allocated only, reference counted.
Definition: QoreListNode.h:52
DLLEXPORT void freeConnectionValues()
frees all connection values
DLLEXPORT QoreStringNode * getPendingDBEncoding() const
returns the pending database-specific character encoding name for the next connection
DLLEXPORT QoreValue getClientVersion(ExceptionSink *xsink) const
executes the "get_client_version" function of the driver, if any, and returns the result
DLLEXPORT void setPendingPassword(const char *p)
sets the password to be used for the next connection
DLLEXPORT bool getAutoCommit() const
returns the autocommit status
DLLEXPORT QoreHashNode * describe(const QoreString *query_str, const QoreListNode *args, ExceptionSink *xsink)
executes SQL that returns a result set and then returns a hash description of the result set
DLLEXPORT QoreValue execRaw(const QoreString *query_str, const QoreListNode *args, ExceptionSink *xsink)
executes SQL throught the "execRaw" function of the DBI driver and returns the result,...
DLLEXPORT void setQoreEncoding(const QoreEncoding *enc)
sets the QoreEncoding used for this connection
Qore's string type supported by the QoreEncoding class.
Definition: QoreString.h:81
DLLEXPORT void connectionRecovered(ExceptionSink *xsink)
should be called be the DBI driver to signify that the connection to the server has been recovered
DLLEXPORT QoreListNode * getCapabilityList() const
returns a QoreListNode object of all capability strings of the current driver, the caller owns the re...
DLLEXPORT void * getPrivateData() const
returns the private DBI-specific data structure for this object
DLLEXPORT bool activeTransaction() const
returns true if a transaction is in progress and DB commands have been issued since the transaction w...
DLLEXPORT void connectionAborted()
should be called by the DBIDriver if the connection to the server is lost
DLLEXPORT void setPendingDBEncoding(const char *c)
sets the database-specific name of the character-encoding to be used for the next connection
const DLLEXPORT char * getDBName() const
returns the database (or schema) name used for the last connection
const DLLEXPORT char * getDriverName() const
returns the name of the current DBI driver
DLLEXPORT QoreValue exec(const QoreString *query_str, const QoreListNode *args, ExceptionSink *xsink)
executes SQL throught the "exec" function of the DBI driver and returns the result,...
DLLEXPORT int beginTransaction(ExceptionSink *xsink)
the implementation of Qore's object data type, reference counted, dynamically-allocated only
Definition: QoreObject.h:61
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
DLLEXPORT QoreStringNode * getConfigString() const
returns a string representing the configuration of the current object
DLLEXPORT QoreHashNode * getOptionHash() const
returns the valid options for this driver with descriptions and current values for the current dataso...
const DLLEXPORT char * getHostName() const
returns the host name used for the last connection
DLLEXPORT QoreStringNode * getPendingPassword() const
returns the pending password for the next connection
DLLEXPORT int rollback(ExceptionSink *xsink)
rolls back the current transaction to the database
DLLLOCAL T & getPrivateDataRef() const
returns the private DBI-specific data structure for this object
Definition: Datasource.h:209
DLLEXPORT int setOption(const char *opt, const QoreValue val, ExceptionSink *xsink)
sets an option for the datasource
DLLEXPORT void setConnectionValues()
copies pending values to current values
DLLEXPORT QoreHashNode * selectRow(const QoreString *query_str, const QoreListNode *args, ExceptionSink *xsink)
executes SQL throught the "selectRow" function of the DBI driver and returns the result,...
DLLEXPORT int beginImplicitTransaction(ExceptionSink *xsink)
calls the "begin_implicit_transaction" DBI method if it exists
DLLEXPORT void connectionLost(ExceptionSink *xsink)
should be called be the DBI driver to signify that the connection to the server has been lost
DLLEXPORT QoreValue getServerVersion(ExceptionSink *xsink)
executes the "get_server_version" function of the driver, if any, and returns the result,...
DLLEXPORT void setPrivateData(void *data)
sets the private DBI-specific data structure for this object
DLLEXPORT int close()
closes the connection
DLLEXPORT bool wasConnectionAborted() const
returns the connection aborted status
DLLEXPORT int open(ExceptionSink *xsink)
opens a connection to the database
the base class for accessing databases in Qore through a Qore DBI driver
Definition: Datasource.h:55
DLLEXPORT QoreValue select(const QoreString *query_str, const QoreListNode *args, ExceptionSink *xsink)
executes SQL throught the "select" function of the DBI driver and returns the result,...
const DLLEXPORT std::string & getHostNameStr() const
returns the host name used for the last connection
defines string encoding functions in Qore
Definition: QoreEncoding.h:83
const DLLEXPORT char * getDBEncoding() const
returns the database-specific character encoding name used for the last connection
const DLLEXPORT std::string & getUsernameStr() const
returns the username used for the last connection
Qore's string value type, reference counted, dynamically-allocated only.
Definition: QoreStringNode.h:50
DLLEXPORT QoreObject * getSQLStatementObjectForResultSet(void *stmt_private_data)
returns an SQLStatement object representing a result set
DLLEXPORT int getPendingPort() const
returns the pending port number for the next connection
DLLEXPORT void setPendingConnectionValues(const Datasource *other)
copies all pending connection values to another Datasource
this class provides the internal link to the database driver for Qore's DBI layer
Definition: DBI.h:350
DLLEXPORT QoreHashNode * getEventQueueHash(Queue *&q, int event_code) const
returns an event hash with only default information in it or 0 if no event queue is set
DLLEXPORT void setTransactionStatus(bool)
sets the transaction status
DLLLOCAL Datasource(DBIDriver *driver, DatasourceStatementHelper *dsh)
creates the object; internal only