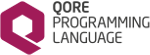 |
Qore Programming Language
0.9.16
|
Go to the documentation of this file.
32 #ifndef _QORE_MODULEMANAGER_H
34 #define _QORE_MODULEMANAGER_H
36 #include <qore/QoreThreadLock.h>
37 #include <qore/QoreString.h>
45 #define QORE_MODULE_API_MAJOR 0
46 #define QORE_MODULE_API_MINOR 24
48 #define QORE_MODULE_COMPAT_API_MAJOR 0
49 #define QORE_MODULE_COMPAT_API_MINOR 24
80 enum mod_op_e { MOD_OP_NONE, MOD_OP_EQ, MOD_OP_GT,
81 MOD_OP_GE, MOD_OP_LT, MOD_OP_LE };
83 typedef std::vector<std::string> strvec_t;
219 static inline bool is_module_api_supported(
int major,
int minor) {
226 #endif // _QORE_MODULEMANAGER_H
void(* qore_module_parse_cmd_t)(const QoreString &cmd, ExceptionSink *xsink)
signature of the module parse command function
Definition: ModuleManager.h:78
void(* qore_module_ns_init_t)(QoreNamespace *root_ns, QoreNamespace *qore_ns)
signature of the module namespace change/delta function
Definition: ModuleManager.h:72
strvec_t dependencies
list of binary modules that this binary module depends on
Definition: ModuleManager.h:105
static DLLEXPORT void addStandardModulePaths()
adds the standard module directories to the module path (only necessary if the module paths are set u...
element of qore_mod_api_list;
Definition: ModuleManager.h:52
supports parsing and executing Qore-language code, reference counted, dynamically-allocated only
Definition: QoreProgram.h:126
This is the hash or associative list container type in Qore, dynamically allocated only,...
Definition: QoreHashNode.h:50
static DLLEXPORT QoreListNode * getModuleList()
retuns a list of module information hashes, caller owns the list reference returned
DLLEXPORT void registerUserModuleFromSource(const char *name, const char *src, QoreProgram *pgm, ExceptionSink *xsink)
registers the given user module from the module source given as an argument
This is the list container type in Qore, dynamically allocated only, reference counted.
Definition: QoreListNode.h:52
contains constants, classes, and subnamespaces in QoreProgram objects
Definition: QoreNamespace.h:65
manages the loading of Qore modules from feature or path names. Also manages adding module changes in...
Definition: ModuleManager.h:122
Qore's string type supported by the QoreEncoding class.
Definition: QoreString.h:81
static DLLEXPORT void addModuleDirList(const char *strlist)
to add a list of directories separated by ':' characters to the QORE_MODULE_DIR list,...
qore_license_t
qore library and module license type identifiers
Definition: common.h:85
static DLLEXPORT void addAutoModuleDir(const char *dir)
no longer supported - removed for security reasons
Qore module info.
Definition: ModuleManager.h:88
const DLLEXPORT qore_mod_api_compat_s * qore_mod_api_list
list of module APIs this library supports
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
static DLLEXPORT void addAutoModuleDirList(const char *strlist)
no longer supported - removed for security reasons
void(* qore_module_delete_t)()
signature of the module destructor function
Definition: ModuleManager.h:75
static DLLEXPORT QoreHashNode * getModuleHash()
retuns a hash of module information hashes, caller owns the list reference returned
QoreHashNode * info
extra information to appear in the module info hash
Definition: ModuleManager.h:108
static DLLEXPORT void addModuleDir(const char *dir)
to add a single directory to the QORE_MODULE_DIR list, can only be called before the library initiali...
void(* qore_binary_module_desc_t)(QoreModuleInfo &mod_info)
Module description function.
Definition: ModuleManager.h:112
static DLLEXPORT QoreStringNode * parseLoadModule(const char *name, QoreProgram *pgm=nullptr)
loads the named module at parse time (or before run time, even if parsing is not active),...
QoreStringNode *(* qore_module_init_t)()
signature of the module constructor/initialization function
Definition: ModuleManager.h:69
const DLLEXPORT unsigned qore_mod_api_list_len
number of elements in qore_mod_api_list;
Qore's string value type, reference counted, dynamically-allocated only.
Definition: QoreStringNode.h:50
DLLEXPORT ModuleManager MM
the global ModuleManager object
static DLLEXPORT int runTimeLoadModule(const char *name, ExceptionSink *xsink)
loads the named module at run time, returns -1 if an exception was raised, 0 for OK