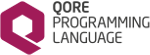 |
Qore Programming Language
0.9.16
|
32 #ifndef QORE_HTTP_CLIENT_OBJECT_H_
33 #define QORE_HTTP_CLIENT_OBJECT_H_
36 #include <qore/QoreSocketObject.h>
37 #include <qore/OutputStream.h>
39 #define HTTPCLIENT_DEFAULT_PORT 80
40 #define HTTPCLIENT_DEFAULT_HOST "localhost"
42 #define HTTPCLIENT_DEFAULT_TIMEOUT 300000
44 #define HTTPCLIENT_DEFAULT_MAX_REDIRECTS 5
54 hashdecl qore_httpclient_priv* http_priv;
63 DLLEXPORT
void lock();
64 DLLEXPORT
void unlock();
121 DLLEXPORT
void addProtocol(
const char* prot,
int port,
bool ssl =
false);
208 DLLEXPORT
void setSecure(
bool is_secure);
255 DLLEXPORT
void sendWithRecvCallback(
const char* meth,
const char* mpath,
const QoreHashNode* headers,
256 const void* data,
unsigned size,
bool getbody,
QoreHashNode* info,
int timeout_ms,
259 DLLEXPORT
void sendWithRecvCallback(
const char* meth,
const char* mpath,
const QoreHashNode* headers,
263 DLLEXPORT
void sendWithCallbacks(
const char* meth,
const char* mpath,
const QoreHashNode* headers,
271 const void* data,
unsigned size,
bool getbody,
QoreHashNode* info,
int timeout_ms,
409 DLLEXPORT
void clearStats();
456 DLLLOCAL
static void static_init();
Interface for private data of output streams.
Definition: OutputStream.h:44
DLLEXPORT QoreHttpClientObject()
creates the QoreHttpClientObject object
const DLLEXPORT char * getDefaultPath() const
returns the default path or 0 if none is set
const DLLEXPORT char * getConnectionPath() const
returns the current connection path or 0 if none is set
DLLEXPORT void setTimeout(int to)
sets the connection and response packet timeout value in milliseconds
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
DLLEXPORT void clearProxyURL()
clears the proxy URL
DLLEXPORT int setProxyURL(const char *proxy, ExceptionSink *xsink)
sets the proxy URL
This is the hash or associative list container type in Qore, dynamically allocated only,...
Definition: QoreHashNode.h:50
DLLEXPORT void addProtocol(const char *prot, int port, bool ssl=false)
adds a protocol
DLLEXPORT QoreHashNode * head(const char *path, const QoreHashNode *headers, QoreHashNode *info, ExceptionSink *xsink)
sends an HTTP "HEAD" method and returns the headers returned, the caller owns the QoreHashNode refere...
virtual DLLEXPORT ~QoreHttpClientObject()
destroys the object and frees all associated memory
DLLEXPORT void setDefaultPort(int prt)
sets the default port
DLLEXPORT AbstractQoreNode * get(const char *path, const QoreHashNode *headers, QoreHashNode *info, ExceptionSink *xsink)
sends an HTTP "GET" method and returns the value of the message body returned, the caller owns the Ab...
DLLEXPORT void clearProxyUserPassword()
clears the username and password for the proxy connection
DLLEXPORT void setConnectTimeout(int ms)
sets the connect timeout in ms
DLLEXPORT bool getEncodingPassthru() const
returns the current encoding_passthru status
DLLEXPORT bool isSecure() const
returns the SSL connection parameter flag
DLLEXPORT AbstractQoreNode * post(const char *path, const QoreHashNode *headers, const void *data, unsigned size, QoreHashNode *info, ExceptionSink *xsink)
sends an HTTP "POST" message to the remote server and returns the message body of the response,...
DLLEXPORT QoreHashNode * getDefaultHeaders() const
Returns a hash of default headers to be sent with every outgoing request.
DLLEXPORT bool getNoDelay() const
returns the value of the TCP_NODELAY flag on the object
DLLEXPORT QoreStringNode * getURL()
returns the connection parameters as a URL, caller owns the reference count returned
DLLEXPORT void setUserPassword(const char *user, const char *pass)
sets the username and password for the connection
DLLEXPORT void setDefaultHeaderValue(const char *header, const char *val)
sets the value of a default header to send with every outgoing message
DLLEXPORT int getConnectTimeout() const
returns the connect timeout in ms, negative numbers mean no timeout
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
DLLEXPORT void sendChunked(const char *meth, const char *mpath, const QoreHashNode *headers, bool getbody, QoreHashNode *info, int timeout_ms, const ResolvedCallReferenceNode *recv_callback, QoreObject *obj, OutputStream *os, InputStream *is, size_t max_chunk_size, const ResolvedCallReferenceNode *trailer_callback, ExceptionSink *xsink)
send a chunked HTTP message through an InputStream and receive the response to an OutputStream
DLLEXPORT void setEncoding(const QoreEncoding *qe)
sets the default encoding for the object
DLLEXPORT void addDefaultHeaders(const QoreHashNode *hdr)
Sets the value of multiple headers to send with every outgoing message.
DLLEXPORT void sendWithOutputStream(const char *meth, const char *mpath, const QoreHashNode *headers, const void *data, unsigned size, bool getbody, QoreHashNode *info, int timeout_ms, const ResolvedCallReferenceNode *recv_callback, QoreObject *obj, OutputStream *os, ExceptionSink *xsink)
make an HTTP request and receive the response to an OutputStream
DLLEXPORT void setMaxRedirects(int max)
sets the max_redirects option
DLLEXPORT void setAssumedEncoding(const char *enc)
sets the assumed encoding
DLLEXPORT void disconnect()
disconnects from the remote server
DLLEXPORT void setDefaultPath(const char *pth)
sets the default path
DLLEXPORT int setNoDelay(bool nodelay)
sets the TCP_NODELAY flag on the object
const DLLEXPORT char * getHTTPVersion() const
returns the http version as a string (either "1.0" or "1.1")
DLLEXPORT void setProxySecure(bool is_secure)
sets the SSL flag for use in the next connection to the proxy
provides a way to communicate with HTTP servers using Qore data structures
Definition: QoreHttpClientObject.h:51
DLLEXPORT bool setEncodingPassthru(bool set)
sets the new and returns the old encoding_passthru status
DLLEXPORT bool isHTTP11() const
returns true if HTTP 1.1 protocol compliance has been set
DLLEXPORT void setHTTP11(bool h11)
sets or clears HTTP 1.1 protocol compliance
DLLEXPORT int setURL(const char *url, ExceptionSink *xsink)
sets the connection URL
the implementation of Qore's object data type, reference counted, dynamically-allocated only
Definition: QoreObject.h:61
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
DLLEXPORT void clearUserPassword()
clears the username and password for the connection
DLLEXPORT bool getErrorPassthru() const
returns the current error_passthru status
DLLEXPORT void setSecure(bool is_secure)
sets the SSL flag for use in the next connection
DLLEXPORT void setProxyUserPassword(const char *user, const char *pass)
sets the username and password for the proxy connection
DLLEXPORT QoreStringNode * getHostHeaderValue() const
returns the Host header value
const DLLEXPORT QoreEncoding * getEncoding() const
returns the default encoding for the object
DLLEXPORT bool setRedirectPassthru(bool set)
sets the new and returns the old redirect_passthru status
DLLEXPORT QoreHashNode * send(const char *meth, const char *path, const QoreHashNode *headers, const void *data, unsigned size, bool getbody, QoreHashNode *info, ExceptionSink *xsink)
sends a message to the remote server and returns the entire response as a hash, caller owns the QoreH...
DLLEXPORT int setHTTPVersion(const char *version, ExceptionSink *xsink)
sets the http version from a string
DLLEXPORT void setConnectionPath(const char *path)
sets or clears the connection path
DLLEXPORT QoreStringNode * getAssumedEncoding() const
gets the assumed encoding
virtual DLLLOCAL void deref()
decrements the reference count of the object without the possibility of throwing a Qore-language exce...
Definition: AbstractPrivateData.h:67
DLLEXPORT int getMaxRedirects() const
returns the value of the max_redirects option
DLLEXPORT bool setErrorPassthru(bool set)
sets the new and returns the old error_passthru status
DLLEXPORT QoreStringNode * getProxyURL()
returns the proxy connection parameters as a URL (or 0 if there is none), caller owns the reference c...
DLLEXPORT void setPersistent(ExceptionSink *xsink)
temporarily disables implicit reconnections; must be called when the server is already connected
base class for resolved call references
Definition: CallReferenceNode.h:105
DLLEXPORT int connect(ExceptionSink *xsink)
opens a connection and returns a code giving the result
defines string encoding functions in Qore
Definition: QoreEncoding.h:83
DLLEXPORT bool getRedirectPassthru() const
returns the current redirect_passthru status
Qore's string value type, reference counted, dynamically-allocated only.
Definition: QoreStringNode.h:50
The base class for all value and parse types in Qore expression trees.
Definition: AbstractQoreNode.h:54
DLLEXPORT int setOptions(const QoreHashNode *opts, ExceptionSink *xsink)
set options with a hash, returns -1 if an exception was thrown, 0 for OK
DLLEXPORT bool isConnected() const
returns the connection status of the object
DLLEXPORT void setEventQueue(Queue *cbq, ExceptionSink *xsink)
sets the event queue, must be already referenced before call
DLLEXPORT bool isProxySecure() const
returns the SSL proxy connection parameter flag
DLLEXPORT int getTimeout() const
returns the connection and response packet timeout value in milliseconds