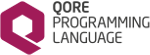 |
Qore Programming Language
0.9.16
|
Go to the documentation of this file.
32 #ifndef _QORE_QORELIB_H
34 #define _QORE_QORELIB_H
37 #include <qore/QoreThreadLock.h>
38 #include <qore/qore_bitopts.h>
39 #include <qore/safe_dslist>
47 #include <sys/types.h>
57 #undef _QORE_HAS_QORE_XMLNODE_CLASS
61 #undef _QORE_HAS_QORE_XMLREADER_CLASS
64 #undef _QORE_HAS_QORE_XMLDOC_CLASS
67 #define _QORE_HAS_HARD_TYPING 1
70 #define _QORE_HAS_DBI_EXECRAW 1
73 #define _QORE_HAS_TIME_ZONES 1
76 #define _QORE_HAS_THREAD_RESOURCE_IDS 1
79 #define _QORE_HAS_PREPARED_STATMENT_API 1
82 #define _QORE_HAS_DATASOURCE_ACTIVETRANSACTION 1
85 #define _QORE_HAS_DBI_SELECT_ROW 1
88 #define _QORE_HAS_NUMBER_TYPE 1
91 #define _QORE_HAS_PATH_IS_READABLE 1
94 #define _QORE_HAS_DBI_OPTIONS 1
97 #define _QORE_HAS_FIND_CREATE_TIMEZONE 1
100 #define _QORE_HAS_NUMBER_CONS_WITH_PREC 1
103 #define _QORE_HAS_FILE_OBJECT_HELPER 1
106 #define _QORE_HAS_QUEUE_OBJECT_HELPER 1
109 #define _QORE_HAS_QOREHTTPCLIENTOBJECT 1
112 #define _QORE_HAS_DBI_DESCRIBE 1
115 #define _QORE_HAS_DBI_EVENTS 1
118 #define _QORE_HAS_QUEUE_OBJECT 1
121 #define _QORE_HAS_SOCKET_PERF_API 1
124 #define _QORE_HAS_QL_MIT 1
127 #define _QORE_HAS_DATETIME_ADD_SECONDS_TO 1
130 #define QCF_NO_FLAGS 0
131 #define QCF_NOOP (1 << 0)
132 #define QCF_USES_EXTRA_ARGS (1 << 1)
133 #define QCF_CONSTANT_INTERN (1 << 2)
134 #define QCF_DEPRECATED (1 << 3)
135 #define QCF_RET_VALUE_ONLY (1 << 4)
136 #define QCF_RUNTIME_NOOP (1 << 5)
137 #define QCF_ABSTRACT_OVERRIDE_ALL (1 << 6)
140 #define QCF_CONSTANT (QCF_CONSTANT_INTERN | QCF_RET_VALUE_ONLY)
159 DLLEXPORT
hashdecl tm*
q_localtime(
const time_t* clock,
struct tm* tms);
162 DLLEXPORT
hashdecl tm*
q_gmtime(
const time_t* clock,
struct tm* tms);
180 DLLEXPORT
char*
q_dirname(
const char* path);
183 DLLEXPORT
void*
q_realloc(
void* ptr,
size_t size);
247 static inline char*
strchrs(
const char* str,
const char* chars) {
249 if (strchr(chars, *str))
257 static inline char*
strnchr(
const char* str,
int len,
char c) {
270 (*str) = tolower(*str);
369 class AbstractQoreZoneInfo;
383 DLLEXPORT
int tz_get_utc_offset(
const AbstractQoreZoneInfo* tz,
int64 epoch_offset,
bool &is_dst,
const char* &zone_name);
385 DLLEXPORT
bool tz_has_dst(
const AbstractQoreZoneInfo* tz);
390 #define QORE_OPT_ATOMIC_OPERATIONS "atomic operations"
391 #define QORE_OPT_STACK_GUARD "stack guard"
393 #define QORE_OPT_SIGNAL_HANDLING "signal handling"
395 #define QORE_OPT_RUNTIME_STACK_TRACE "runtime stack tracing"
397 #define QORE_OPT_LIBRARY_DEBUGGING "library debugging"
399 #define QORE_OPT_SHA "openssl sha"
401 #define QORE_OPT_SHA224 "openssl sha224"
403 #define QORE_OPT_SHA256 "openssl sha256"
405 #define QORE_OPT_SHA384 "openssl sha384"
407 #define QORE_OPT_SHA512 "openssl sha512"
409 #define QORE_OPT_MDC2 "openssl mdc2"
411 #define QORE_OPT_RC5 "openssl rc5"
413 #define QORE_OPT_MD2 "openssl md2"
415 #define QORE_OPT_DSS "openssl dss"
417 #define QORE_OPT_TERMIOS "termios"
419 #define QORE_OPT_FILE_LOCKING "file locking"
421 #define QORE_OPT_UNIX_USERMGT "unix user management"
423 #define QORE_OPT_UNIX_FILEMGT "unix file management"
425 #define QORE_OPT_DETERMINISTIC_GC "deterministic GC"
427 #define QORE_OPT_FUNC_ROUND "round()"
429 #define QORE_OPT_FUNC_TIMEGM "timegm()"
431 #define QORE_OPT_FUNC_SETEUID "seteuid()"
433 #define QORE_OPT_FUNC_SETEGID "setegid()"
435 #define QORE_OPT_FUNC_SYSTEM "system()"
437 #define QORE_OPT_FUNC_KILL "kill()"
439 #define QORE_OPT_FUNC_FORK "fork()"
441 #define QORE_OPT_FUNC_GETPPID "getppid()"
443 #define QORE_OPT_FUNC_STATVFS "statvfs()"
445 #define QORE_OPT_FUNC_SETSID "setsid()"
447 #define QORE_OPT_FUNC_IS_EXECUTABLE "is_executable()"
449 #define QORE_OPT_FUNC_CLOSE_ALL_FD "close_all_fd()"
451 #define QORE_OPT_FUNC_GET_NETIF_LIST "get_netif_list()"
456 #define QO_ALGORITHM 1
457 #define QO_FUNCTION 2
533 #define QORE_MAX(a, b) ((a) > (b) ? (a) : (b))
536 #define QORE_MIN(a, b) ((a) < (b) ? (a) : (b))
539 #define QORE_PARAM_NO_ARG QoreSimpleValue().assign(nullptr)
542 #ifndef QORE_PATH_MAX
543 #ifdef _XOPEN_PATH_MAX
544 #define QORE_PATH_MAX _XOPEN_PATH_MAX
546 #define QORE_PATH_MAX 1024
601 DLLEXPORT
void*
q_memmem(
const void* big,
size_t big_len,
const void* little,
size_t little_len);
608 DLLEXPORT
void*
q_memrmem(
const void* big,
size_t big_len,
const void* little,
size_t little_len);
620 DLLEXPORT
double q_strtod(
const char* str);
746 #endif // _QORE_QORELIB_H
definition of the elements in the qore_option_list
Definition: QoreLib.h:460
DLLEXPORT char * q_basenameptr(const char *path)
returns a pointer within the same string
static void strtolower(char *str)
convert a string to lower-case in place
Definition: QoreLib.h:268
static char * strnchr(const char *str, int len, char c)
find a character in a string up to len
Definition: QoreLib.h:257
DLLEXPORT QoreListNode * domain_bitfield_to_string_list(int64 i, ExceptionSink *xsink)
returns a list<string> of domain strings for the given bitfield; a Qore-language exception is raised ...
DLLEXPORT QoreValue qore_get_module_option(std::string mod, std::string opt)
get module option for the given module
DLLEXPORT bool q_parse_bool(QoreValue n)
tries to parse a boolean value - standard conversion or uses q_parse_bool(const char*) if it's a stri...
std::vector< int > sig_vec_t
signal vector
Definition: QoreLib.h:51
DLLEXPORT FeatureList qoreFeatureList
list of qore features
supports parsing and executing Qore-language code, reference counted, dynamically-allocated only
Definition: QoreProgram.h:126
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
const DLLEXPORT char * tz_get_region_name(const AbstractQoreZoneInfo *tz)
returns the reion name for the given time zone
virtual const DLLEXPORT char * getTypeName() const =0
returns the type name as a c string
This is the hash or associative list container type in Qore, dynamically allocated only,...
Definition: QoreHashNode.h:50
DLLEXPORT int qore_release_signal(int sig, const char *name)
releases the signal allocated to the given module
const DLLEXPORT AbstractQoreZoneInfo * findCreateOffsetZone(int seconds_east)
returns a time zone for the given time zone UTC offset
const char * constant
name of the constant for this option
Definition: QoreLib.h:462
DLLEXPORT void qore_set_module_option(std::string mod, std::string opt, QoreValue val)
sets a module option for the given module
DLLEXPORT QoreStringNode * qore_bunzip2_to_string(const BinaryNode *bin, const QoreEncoding *enc, ExceptionSink *xsink)
decompresses bzip2 data to a string
DLLEXPORT void qore_setup_argv(int pos, int argc, char *argv[])
sets up the Qore ARGV and QORE_ARGV values
DLLEXPORT bool q_path_is_readable(const char *path)
platform-independent API that tells if the given path is readable by the current user
DLLEXPORT double q_strtod(const char *str)
converts a string to a double in a locale-independent way
const qore_type_t NT_NOTHING
type value for QoreNothingNode
Definition: node_types.h:42
int type
the type of the option
Definition: QoreLib.h:463
DLLEXPORT void * q_memrmem(const void *big, size_t big_len, const void *little, size_t little_len)
finds a memory sequence in a larger memory sequence searching from the end of the sequence
defines a Qore-language class
Definition: QoreClass.h:239
DLLEXPORT char * q_dirname(const char *path)
thread-safe dirname function (resulting pointer must be free()ed)
DLLEXPORT bool q_absolute_path_unix(const char *path)
returns true if the given string is an absolute path on UNIX systems
DLLEXPORT void qore_disable_gc()
this function will cause garbage collection to be disabled
DLLEXPORT int64 getMsZeroBigInt(QoreValue a)
for getting an integer number of milliseconds, with 0 as the default, from either a relative time val...
DLLLOCAL qore_type_t getType() const
returns the data type
Definition: AbstractQoreNode.h:172
DLLEXPORT int64 getSecZeroBigInt(QoreValue a)
for getting an integer number of seconds, with 0 as the default, from either a relative time value or...
This is the list container type in Qore, dynamically allocated only, reference counted.
Definition: QoreListNode.h:52
DLLEXPORT int q_env_subst(QoreString &str)
performs environment variable substitution on the string argument
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
DLLEXPORT QoreListNode * parse_option_bitfield_to_string_list(int64 i, ExceptionSink *xsink)
returns a list<string> of parse option strings for the given bitfield; a Qore-language exception is r...
DLLEXPORT QoreHashNode * q_get_thread_local_vars(int frame, ExceptionSink *xsink)
retrieves a hash of all thread local variables and their values for the given stack frame in the curr...
DLLEXPORT int q_get_data(const QoreValue &data, const char *&ptr, size_t &len)
returns the pointer and size for string or binary data (return 0); no change for other data (return -...
DLLEXPORT BinaryNode * qore_bzip2(void *ptr, unsigned long len, int level, ExceptionSink *xsink)
compresses data with bzip2
int q_uname2uid(const char *name, uid_t &uid)
thread-safe way to lookup a uid from a username
DLLEXPORT int64 q_epoch_ns(int &us)
returns the seconds and nanoseconds from the epoch
DLLEXPORT void qore_apply_rounding_heuristic(QoreString &str, int round_threshold_1, int round_threshold_2)
DLLEXPORT void parse_set_time_zone(const char *zone)
to set the time zone from the command line
DLLEXPORT size_t q_thread_get_stack_size()
returns the default thread stack size
DLLEXPORT BinaryNode * parseHex(const char *buf, int len, ExceptionSink *xsink)
parses a string of hex characters and returns a BinaryNode
Qore's string type supported by the QoreEncoding class.
Definition: QoreString.h:81
DLLEXPORT BinaryNode * parseBase64(const char *buf, int len, ExceptionSink *xsink)
parses a string of base64-encoded data and returns a BinaryNode
DLLEXPORT int getSecMinusOneInt(QoreValue a)
for getting an integer number of seconds, with -1 as the default, from either a relative time value o...
DLLEXPORT size_t q_thread_stack_remaining()
Returns the number of bytes left in the current thread stack.
DLLEXPORT qore_license_t qore_get_license()
returns the license type that the library has been initialized under
qore_license_t
qore library and module license type identifiers
Definition: common.h:85
DLLEXPORT BinaryNode * qore_gzip(void *ptr, unsigned long len, int level, ExceptionSink *xsink)
gzips data
DLLEXPORT int q_realpath(const QoreString &path, QoreString &rv, ExceptionSink *xsink=0)
normalizes the given path and resolves any symlinks
DLLLOCAL FeatureList()
initialized by the library, constructor not exported
static bool is_nothing(const AbstractQoreNode *n)
to check if an AbstractQoreNode object is NOTHING
Definition: QoreLib.h:316
DLLEXPORT void * q_memmem(const void *big, size_t big_len, const void *little, size_t little_len)
finds a memory sequence in a larger memory sequence
DLLEXPORT int getSecZeroInt(QoreValue a)
for getting an integer number of seconds, with 0 as the default, from either a relative time value or...
DLLEXPORT bool qore_is_gc_enabled()
returns true if garbage collection is enabled, false if not
STL-like list containing all presently-loaded Qore features.
Definition: QoreLib.h:227
DLLEXPORT BinaryNode * qore_gunzip_to_binary(const BinaryNode *bin, ExceptionSink *xsink)
gunzips compressed data to a binary
DLLEXPORT QoreStringNode * qore_reassign_signals(const sig_vec_t &sig_vec, const char *name)
allows a module to take over ownership of multiple signals atomically
DLLEXPORT bool q_absolute_path_windows(const char *path)
returns true if the given string is an absolute path on Windows systems
DLLEXPORT int getMicroSecZeroInt(QoreValue a)
for getting an integer number of microseconds, with 0 as the default, from either a relative time val...
DLLEXPORT QoreHashNode * q_getpwuid(uid_t uid)
thread-safe version of getpwuid(): returns a Qore hash of the passwd information from the uid if poss...
DLLEXPORT int64 getMicroSecZeroInt64(QoreValue a)
for getting an integer number of microseconds, with 0 as the default, from either a relative time val...
DLLEXPORT QoreStringNode * q_strerror(int errnum)
returns the error string as a QoreStringNode
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
DLLEXPORT BinaryNode * qore_deflate(void *ptr, unsigned long len, int level, ExceptionSink *xsink)
compresses data with the DEFLATE algorithm
DLLEXPORT size_t qore_option_list_size
number of elements in the option list
DLLEXPORT char * q_basename(const char *path)
thread-safe basename function (resulting pointer must be free()ed)
DLLEXPORT bool tz_has_dst(const AbstractQoreZoneInfo *tz)
returns true if the zone has daylight savings time ever
DLLEXPORT int getMsZeroInt(QoreValue a)
for getting an integer number of milliseconds, with 0 as the default, from either a relative time val...
const DLLEXPORT char * get_full_type_name(const AbstractQoreNode *n)
returns a string type description of the full type of the value contained (ex: "nothing" for a null A...
DLLEXPORT QoreStringNode * q_vsprintf(const QoreListNode *params, int field, int offset, ExceptionSink *xsink)
a string formatting function that works with Qore data structures
DLLEXPORT QoreProgram * qore_get_call_program_context()
returns the caller's Program context, if any
int q_gname2gid(const char *name, gid_t &gid)
thread-safe way to lookup a gid from a group name
DLLEXPORT QoreHashNode * q_getgrgid(uid_t uid)
thread-safe version of getgrgid(): returns a Qore hash of the group information from the gid if possi...
DLLEXPORT bool q_libqore_exiting()
returns true if the Qore library is exiting without a shutdown
DLLEXPORT QoreStringNode * qore_inflate_to_string(const BinaryNode *b, const QoreEncoding *enc, ExceptionSink *xsink)
decompresses data compressed with the DEFLATE algorithm to a string
DLLEXPORT int64 q_epoch_us(int &us)
returns the seconds and microseconds from the epoch
DLLEXPORT int64 getSecMinusOneBigInt(QoreValue a)
for getting an integer number of seconds, with -1 as the default, from either a relative time value o...
const DLLEXPORT char * q_mode_to_perm(mode_t mode, QoreString &perm)
concatenates UNIX-style permissions to perm and from mode and returns a string giving the file type
DLLEXPORT QoreStringNode * q_sprintf(const QoreListNode *params, int field, int offset, ExceptionSink *xsink)
a string formatting function that works with Qore data structures
DLLEXPORT bool q_get_option_constant_value(const char *opt)
returns the boolean value of qore library the given name of the constant for the option; false if the...
DLLEXPORT int qore_usleep(int64 usecs)
use this function instead of usleep(), as usleep() is not signal-safe on some platforms (ex: Solaris ...
DLLEXPORT QoreStringNode * qore_gunzip_to_string(const BinaryNode *bin, const QoreEncoding *enc, ExceptionSink *xsink)
gunzips compressed data to a string
DLLEXPORT hashdecl tm * q_gmtime(const time_t *clock, hashdecl tm *tms)
thread-safe version of "gmtime()"
DLLEXPORT QoreHashNode * q_getpwnam(const char *name)
thread-safe version of getpwnam(): returns a Qore hash of the passwd information from the username if...
DLLEXPORT void qore_exit_process(int rc)
instead of calling "exit()", call qore_exit_process() to exit without risking a crash if other thread...
const DLLEXPORT QoreClass * qore_pseudo_get_class(qore_type_t t)
returns the pseudo-class for the given type
DLLEXPORT size_t q_thread_set_stack_size(size_t size, ExceptionSink *xsink)
sets the default thread stack size or throws an exception on error
DLLEXPORT int64 getMsMinusOneBigInt(QoreValue a)
for getting an integer number of milliseconds, with -1 as the default, from either a relative time va...
static char * strchrs(const char *str, const char *chars)
find one of any characters in a string
Definition: QoreLib.h:247
DLLEXPORT char * make_class_name(const char *fn)
function to try and make a class name out of a file path, returns a new string that must be free()ed
bool value
the value of the option
Definition: QoreLib.h:464
DLLEXPORT void deref(ExceptionSink *xsink)
decrements the reference count and calls derefImpl() if there_can_be_only_one is false,...
DLLEXPORT int q_set_thread_var_value(int frame, const char *name, const QoreValue &val, ExceptionSink *xsink)
sets the value of the given thread-local variable (which may be a closure-bound variable as well) in ...
DLLEXPORT bool q_libqore_shutdown()
returns true if the Qore library has been shut down
const DLLEXPORT QoreTypeInfo * get_or_nothing_type_check(const QoreTypeInfo *typeInfo)
returns the "or nothing" type for the given type
DLLEXPORT bool q_get_option_value(const char *opt)
returns the boolean value of qore library the given option name; false if the option is unknown
DLLEXPORT int64 q_epoch()
returns the seconds from the epoch
DLLEXPORT int qore_release_signals(const sig_vec_t &sig_vec, const char *name)
releases multiple signals allocated to the given module atomically
const char * option
name of the option
Definition: QoreLib.h:461
DLLEXPORT QoreStringNode * qore_reassign_signal(int sig, const char *name)
allows a module to take over ownership of a signal
DLLEXPORT int getMsMinusOneInt(QoreValue a)
for getting an integer number of milliseconds, with -1 as the default, from either a relative time va...
DLLEXPORT BinaryNode * qore_inflate_to_binary(const BinaryNode *b, ExceptionSink *xsink)
decompresses data compressed with the DEFLATE algorithm to a binary
defines string encoding functions in Qore
Definition: QoreEncoding.h:83
holds arbitrary binary data
Definition: BinaryNode.h:41
DLLEXPORT size_t q_thread_stack_used()
Returns the number of bytes used in the current thread stack.
static char * strtoupper(char *str)
convert a string to upper-case in place
Definition: QoreLib.h:276
const DLLEXPORT qore_option_s * qore_option_list
list of qore options
DLLEXPORT BinaryNode * qore_bunzip2_to_binary(const BinaryNode *bin, ExceptionSink *xsink)
decompresses bzip2 data to a binary
DLLEXPORT hashdecl tm * q_localtime(const time_t *clock, hashdecl tm *tms)
thread-safe version of "localtime()"
Qore's string value type, reference counted, dynamically-allocated only.
Definition: QoreStringNode.h:50
DLLEXPORT int tz_get_utc_offset(const AbstractQoreZoneInfo *tz, int64 epoch_offset, bool &is_dst, const char *&zone_name)
returns the UTC offset and local time zone name for the given time given as seconds from the epoch (1...
The base class for all value and parse types in Qore expression trees.
Definition: AbstractQoreNode.h:54
int q_getcwd(QoreString &cwd)
returns the current working directory in the given string; -1 is returned if an error occurred,...
int16_t qore_type_t
used to identify unique Qore data and parse types (descendents of AbstractQoreNode)
Definition: common.h:70
const DLLEXPORT AbstractQoreZoneInfo * find_create_timezone(const char *name, ExceptionSink *xsink)
returns a time zone for the given region name or UTC offset given as a string ("+01:00")
DLLLOCAL ~FeatureList()
destructor not exported
static void discard(AbstractQoreNode *n, ExceptionSink *xsink)
to deref an AbstractQoreNode (when the pointer may be 0)
Definition: QoreLib.h:324
DLLEXPORT void * q_realloc(void *ptr, size_t size)
frees memory if there is an allocation error
DLLEXPORT bool q_absolute_path(const char *path)
returns true if the given string is an absolute path on the current platform
DLLEXPORT void q_normalize_path(QoreString &path, const char *cwd=0)
normalizes the given path for the current platform in place (makes absolute, removes "....
DLLEXPORT QoreHashNode * q_getgrnam(const char *name)
thread-safe version of getgrnam(): returns a Qore hash of the group information from the group name i...
DLLEXPORT bool q_libqore_initalized()
returns true if the Qore library has been initialized