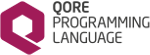 |
Qore Programming Language
0.9.16
|
42 #ifndef _QORE_QORENAMESPACE_H
44 #define _QORE_QORENAMESPACE_H
51 class QoreExternalFunction;
53 class QoreExternalGlobalVar;
66 friend class QoreNamespaceList;
68 friend class qore_ns_private;
69 friend class qore_root_ns_private;
70 friend hashdecl NSOInfoBase;
162 DLLEXPORT
const char*
getName()
const;
257 const QoreTypeInfo* returnTypeInfo =
nullptr,
unsigned num_params = 0, ...);
284 DLLEXPORT std::string
getPath(
bool anchored =
false)
const;
304 DLLEXPORT
bool isRoot()
const;
324 class qore_ns_private* priv;
336 friend class qore_ns_private;
337 friend class qore_root_ns_private;
338 friend class StaticSystemNamespace;
357 class qore_root_ns_private* rpriv;
369 class QorePrivateNamespaceIterator;
383 DLLEXPORT
bool next();
409 QorePrivateNamespaceIterator* priv;
424 DLLEXPORT
bool next();
442 QorePrivateNamespaceIterator* priv;
457 DLLEXPORT
bool next();
469 class qore_namespace_namespace_iterator* priv;
484 DLLEXPORT
bool next();
487 DLLEXPORT
const QoreExternalFunction&
get()
const;
496 class qore_namespace_function_iterator* priv;
511 DLLEXPORT
bool next();
523 class qore_namespace_constant_iterator* priv;
538 DLLEXPORT
bool next();
550 class ConstClassListIterator* priv;
565 DLLEXPORT
bool next();
568 DLLEXPORT
const QoreExternalGlobalVar&
get()
const;
577 class qore_namespace_globalvar_iterator* priv;
592 DLLEXPORT
bool next();
604 class ConstHashDeclListIterator* priv;
607 #endif // QORE_NAMESPACE_H
DLLEXPORT QoreNamespaceClassIterator(const QoreNamespace &ns)
creates the iterator
QoreValue(* q_external_func_t)(const void *ptr, const QoreListNode *args, q_rt_flags_t flags, ExceptionSink *xsink)
the type used for builtin function signatures for external functions
Definition: common.h:319
DLLEXPORT void addSystemHashDecl(TypedHashDecl *hashdecl)
adds a hashdecl to a namespace
#define PO_DEFAULT
no parse options set by default
Definition: Restrictions.h:106
DLLEXPORT QoreClass * findLoadLocalClass(const char *cname)
finds a class in this namespace, does not search child namespaces
DLLEXPORT bool isBuiltin() const
returns true if the namespace is builtin
virtual DLLEXPORT ~QoreNamespaceTypedHashIterator()
destroys the object
DLLEXPORT bool next()
moves to the next position; returns true if on a valid position
QoreValue(* q_func_n_t)(const QoreListNode *args, q_rt_flags_t flags, ExceptionSink *xsink)
the type used for builtin function signatures
Definition: common.h:307
allows typed hashes (hashdecls) in a namespace to be iterated
Definition: QoreNamespace.h:583
supports parsing and executing Qore-language code, reference counted, dynamically-allocated only
Definition: QoreProgram.h:126
const DLLEXPORT QoreNamespace * operator*() const
returns the namespace
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
#define QDOM_DEFAULT
the default domain (no domain)
Definition: Restrictions.h:156
This is the hash or associative list container type in Qore, dynamically allocated only,...
Definition: QoreHashNode.h:50
virtual DLLEXPORT ~QoreNamespaceIterator()
destroys the object
DLLEXPORT bool next()
moves to the next position; returns true if on a valid position
DLLEXPORT QoreNamespaceConstIterator(const QoreNamespace &ns)
creates the iterator; the namespace given will also be included in the iteration set
allows functions in a namespace to be iterated
Definition: QoreNamespace.h:475
const DLLEXPORT QoreClass & get() const
returns the class
virtual DLLEXPORT ~QoreNamespaceClassIterator()
destroys the object
defines a Qore-language class
Definition: QoreClass.h:239
DLLEXPORT void addConstant(const char *name, QoreValue value)
adds a constant definition to the namespace
DLLEXPORT void addBuiltinVariant(const char *name, q_func_n_t f, int64 code_flags=QCF_NO_FLAGS, int64 functional_domain=QDOM_DEFAULT, const QoreTypeInfo *returnTypeInfo=0, unsigned num_params=0,...)
adds a function variant
const DLLEXPORT QoreExternalFunction & get() const
returns the function
virtual DLLEXPORT ~QoreNamespaceConstIterator()
destroys the object
DLLEXPORT QoreHashNode * getInfo() const
returns a hash giving information about the definitions in the namespace
const DLLEXPORT TypedHashDecl & get() const
returns the typed hash (hashdecl)
DLLEXPORT QoreClass * findLocalClass(const char *cname) const
finds a class in this namespace, does not search child namespaces
DLLEXPORT std::string getPath(bool anchored=false) const
returns the path for the namespace
DLLEXPORT QoreNamespaceConstantIterator(const QoreNamespace &ns)
creates the iterator
const DLLEXPORT QoreNamespace & get() const
returns the namespace
const DLLEXPORT QoreNamespace * getParent() const
returns a pointer to the parent namespace or nullptr if there is no parent
DLLEXPORT QoreNamespace * copy(int po) const
returns a deep copy of the namespace; DEPRECATED: use copy(int64) instead
DLLEXPORT QoreNamespaceIterator(QoreNamespace &ns)
creates the iterator; the namespace given will also be included in the iteration set
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
DLLEXPORT QoreNamespaceNamespaceIterator(const QoreNamespace &ns)
creates the iterator
DLLEXPORT QoreNamespace * operator*()
returns the namespace
DLLEXPORT QoreNamespace * operator->()
returns the namespace
const DLLEXPORT char * getModuleName() const
Returns the module name the class was loaded from or nullptr if it is a builtin namespace.
allows all namespaces of a namespace to be iterated (including the namespace passed in the constructo...
Definition: QoreNamespace.h:415
const DLLEXPORT TypedHashDecl * findLocalTypedHash(const char *name) const
find a typed hash (hashdecl) in the current namespace; returns nullptr if not found
const DLLEXPORT QoreExternalGlobalVar * findLocalGlobalVar(const char *name) const
find a global variable in the current namespace; returns nullptr if not found
DLLEXPORT QoreProgram * getProgram() const
Returns the owning QoreProgram object (if not the static system namespace)
contains constants, classes, and subnamespaces in QoreProgram objects
Definition: QoreNamespace.h:65
DLLEXPORT QoreNamespace * findLocalNamespace(const char *nsname) const
finds a subnamespace in this namespace, does not search child namespaces
const DLLEXPORT QoreExternalGlobalVar & get() const
returns the global variable
external wrapper class for constants
Definition: QoreReflection.h:200
DLLEXPORT QoreNamespace * rootGetQoreNamespace() const
returns a pointer to the QoreNamespace for the "Qore" namespace
virtual DLLEXPORT ~QoreNamespace()
destroys the object and frees memory
DLLEXPORT void deleteData(ExceptionSink *xsink)
this function must be called before the QoreNamespace object is deleted or a crash could result due i...
const DLLEXPORT char * getName() const
returns the name of the namespace
DLLEXPORT bool next()
moves to the next position; returns true if on a valid position
virtual DLLLOCAL ~RootQoreNamespace()
destructor is not exported in the library's public API
DLLEXPORT bool isRoot() const
returns true if the namespace is the root namespace
DLLEXPORT bool next()
moves to the next position; returns true if on a valid position
typed hash declaration
Definition: TypedHashDecl.h:44
DLLEXPORT QoreNamespaceFunctionIterator(const QoreNamespace &ns)
creates the iterator
DLLEXPORT QoreNamespaceGlobalVarIterator(const QoreNamespace &ns)
creates the iterator
DLLEXPORT QoreNamespaceTypedHashIterator(const QoreNamespace &ns)
creates the iterator
DLLEXPORT bool next()
moves to the next position; returns true if on a valid position
const DLLEXPORT QoreNamespace & get() const
returns the namespace
DLLEXPORT QoreNamespace & get()
returns the namespace
DLLEXPORT QoreHashNode * getConstantInfo() const
a hash of all constants in the namespace, the hash keys are the constant names and the values are the...
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
virtual DLLEXPORT ~QoreNamespaceFunctionIterator()
destroys the object
DLLEXPORT void setClassHandler(q_ns_class_handler_t class_handler)
sets the namespace class handler
allows classes in a namespace to be iterated
Definition: QoreNamespace.h:529
allows local namespaces to be iterated
Definition: QoreNamespace.h:448
virtual DLLEXPORT ~QoreNamespaceNamespaceIterator()
destroys the object
const DLLEXPORT QoreExternalConstant * findLocalConstant(const char *name) const
find a constant in the current namespace; returns nullptr if not found
const DLLEXPORT QoreExternalConstant & get() const
returns the constant
DLLEXPORT void addNamespace(QoreNamespace *ns)
adds a namespace to the namespace tree
virtual DLLEXPORT ~QoreNamespaceConstantIterator()
destroys the object
const DLLEXPORT QoreExternalFunction * findLocalFunction(const char *name) const
find a function in the current namespace; returns nullptr if not found
DLLEXPORT QoreProgram * getProgram() const
Returns the owning QoreProgram object (if not the static system namespace)
virtual DLLEXPORT ~QoreNamespaceGlobalVarIterator()
destroys the object
allows global variables in a namespace to be iterated
Definition: QoreNamespace.h:556
DLLEXPORT QoreNamespace * findCreateNamespacePathAll(const char *nspath)
finds a Namespace based on the argument; creates it (or the whole path) if necessary
const DLLEXPORT QoreNamespace * operator->() const
returns the namespace
DLLEXPORT bool isModulePublic() const
returns true if the namespace has its module public flag set
DLLEXPORT QoreHashNode * getClassInfo() const
gets a hash of all classes in the namespace, the hash keys are the class names and the values are lis...
static unsigned num_params(const QoreListNode *n)
returns the number of arguments passed to the function
Definition: params.h:54
DLLEXPORT void addInitialNamespace(QoreNamespace *ns)
adds a subnamespace to the namespace
allows constants in a namespace to be iterated
Definition: QoreNamespace.h:502
DLLEXPORT bool isImported() const
returns true if the namespace was imported from another program object
DLLEXPORT QoreNamespace * findCreateNamespacePath(const char *nspath)
finds a Namespace based on the argument; creates it (or the whole path) if necessary
the root namespace of a QoreProgram object
Definition: QoreNamespace.h:335
allows all namespaces of a namespace to be iterated (including the namespace passed in the constructo...
Definition: QoreNamespace.h:374
DLLEXPORT bool next()
moves to the next position; returns true if on a valid position
DLLEXPORT void clear(ExceptionSink *xsink)
clears the contents of the namespace before deleting
DLLEXPORT bool next()
moves to the next position; returns true if on a valid position
DLLEXPORT QoreNamespace(const char *n)
creates a namespace with the given name
DLLEXPORT bool next()
moves to the next position; returns true if on a valid position
DLLEXPORT void addSystemClass(QoreClass *oc)
adds a class to a namespace