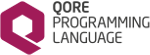 |
Qore Programming Language
0.9.16
|
32 #ifndef _QORE_QORENUMBERNODE_H
34 #define _QORE_QORENUMBERNODE_H
42 #define QORE_NF_DEFAULT 0
44 #define QORE_NF_SCIENTIFIC (1 << 0)
46 #define QORE_NF_RAW (1 << 1)
52 friend hashdecl qore_number_private;
56 DLLLOCAL
virtual bool getAsBoolImpl()
const;
59 DLLLOCAL
virtual int getAsIntImpl()
const;
62 DLLLOCAL
virtual int64 getAsBigIntImpl()
const;
65 DLLLOCAL
virtual double getAsFloatImpl()
const;
69 hashdecl qore_number_private*
priv;
213 DLLEXPORT
bool zero()
const;
216 DLLEXPORT
int sign()
const;
222 DLLEXPORT
bool lessThan(
double n)
const;
258 DLLEXPORT
bool equals(
double n)
const;
273 DLLEXPORT
unsigned getPrec()
const;
276 DLLEXPORT
bool nan()
const;
279 DLLEXPORT
bool inf()
const;
285 DLLLOCAL
virtual void parseInit(
QoreValue& val, LocalVar *oflag,
int pflag,
int &lvids,
const QoreTypeInfo *&returnTypeInfo);
317 DLLLOCAL
void*
operator new(size_t);
const qore_type_t NT_NUMBER
type value for QoreNumberNode
Definition: node_types.h:53
static const DLLLOCAL char * getStaticTypeName()
returns the type name (useful in templates)
Definition: QoreNumberNode.h:297
DLLEXPORT bool greaterThanOrEqual(const QoreNumberNode &n) const
returns true if the current object is greater than or equal to the argument
virtual DLLEXPORT int getAsString(QoreString &str, int foff, class ExceptionSink *xsink) const
concatenate the string representation of the number value to an existing QoreString
virtual DLLEXPORT AbstractQoreNode * realCopy() const
returns a copy of the object; the caller owns the reference count
DLLLOCAL bool is_temp() const
returns true if the referenced being managed is temporary
Definition: QoreNumberNode.h:346
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
DLLEXPORT QoreNumberNode * doMinus(const QoreNumberNode &n) const
subtract the argument from this value and return the result
DLLEXPORT unsigned getPrec() const
returns the precision of the number
virtual DLLEXPORT QoreString * getStringRepresentation(bool &del) const
returns the number value converted to a string and sets del to true
virtual DLLEXPORT bool is_equal_soft(const AbstractQoreNode *v, ExceptionSink *xsink) const
tests for equality with possible type conversion (soft compare)
hashdecl qore_number_private * priv
the private implementation of the type
Definition: QoreNumberNode.h:69
const DLLLOCAL QoreNumberNode * operator->()
returns the object being managed
Definition: QoreNumberNode.h:330
DLLLOCAL ~QoreNumberNodeHelper()
destroys the object and dereferences the pointer being managed if it was a temporary object
Qore's arbitrary-precision number value type, dynamically-allocated only, reference counted.
Definition: QoreNumberNode.h:51
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
static DLLEXPORT QoreNumberNode * toNumber(const AbstractQoreNode *v)
returns the representation of the value as a number if possible (otherwise returns 0),...
Qore's string type supported by the QoreEncoding class.
Definition: QoreString.h:81
DLLEXPORT bool lessThan(const QoreNumberNode &n) const
returns true if the current object is less than the argument
manages conversions of a QoreValue to a QoreNumberNode
Definition: QoreNumberNode.h:310
DLLEXPORT QoreNumberNode * getReferencedValue()
returns a referenced value and leaves the current object empty; the caller will own the reference
static DLLLOCAL qore_type_t getStaticTypeCode()
returns the type code (useful in templates)
Definition: QoreNumberNode.h:302
virtual DLLLOCAL void parseInit(QoreValue &val, LocalVar *oflag, int pflag, int &lvids, const QoreTypeInfo *&returnTypeInfo)
returns the type information
DLLEXPORT bool equals(const QoreNumberNode &n) const
returns true if the current object is equal to the argument
virtual const DLLEXPORT char * getTypeName() const
returns the type name as a c string
DLLEXPORT QoreNumberNode()
creates a new numbering-point value and assigns it to 0
DLLEXPORT bool lessThanOrEqual(const QoreNumberNode &n) const
returns true if the current object is less than or equal to the argument
virtual DLLEXPORT class DateTime * getDateTimeRepresentation(bool &del) const
returns the DateTime representation of this value and sets del to true
base class for simple value types
Definition: AbstractQoreNode.h:366
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
DLLEXPORT int sign() const
returns -1 if the number is negative, 0 if zero, or 1 if the number is positive
DLLEXPORT QoreNumberNode * doDivideBy(const QoreNumberNode &n, ExceptionSink *xsink) const
divide this value by the argument return the result (can throw a division-by-zero exception)
DLLEXPORT bool zero() const
returns true if the number is zero
virtual DLLEXPORT ~QoreNumberNode()
the destructor is protected because it should not be called directly
DLLEXPORT void toString(QoreString &str, int fmt=QORE_NF_DEFAULT) const
concatenates the string value corresponding to the number to the string given
Holds absolute and relative date/time values in Qore with precision to the microsecond.
Definition: DateTime.h:93
DLLEXPORT bool inf() const
returns true if the number is +/-inf
DLLEXPORT bool ordinary() const
returns true if the number is an ordinary number (neither NaN nor an infinity)
DLLEXPORT QoreNumberNode * doMultiply(const QoreNumberNode &n) const
multiply the argument to this value and return the result
virtual DLLEXPORT bool is_equal_hard(const AbstractQoreNode *v, ExceptionSink *xsink) const
tests for equality without type conversions (hard compare)
DLLEXPORT QoreNumberNode * negate() const
returns the negative of the current object (this)
const DLLLOCAL QoreNumberNode * operator*()
returns the object being managed
Definition: QoreNumberNode.h:336
DLLEXPORT QoreNumberNode * numberRefSelf() const
returns a pointer to this with the reference count incremented
The base class for all value and parse types in Qore expression trees.
Definition: AbstractQoreNode.h:54
int16_t qore_type_t
used to identify unique Qore data and parse types (descendents of AbstractQoreNode)
Definition: common.h:70
DLLEXPORT bool nan() const
returns true if the number is NaN
DLLEXPORT QoreNumberNode * doPlus(const QoreNumberNode &n) const
add the argument to this value and return the result
DLLEXPORT bool greaterThan(const QoreNumberNode &n) const
returns true if the current object is greater than the argument