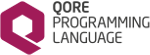 |
Qore Programming Language
0.9.16
|
34 #ifndef _QORE_QOREPROGRAM_H
36 #define _QORE_QOREPROGRAM_H
38 #include <qore/AbstractPrivateData.h>
44 #define QP_WARN_NONE 0
45 #define QP_WARN_WARNING_MASK_UNCHANGED (1 << 0)
46 #define QP_WARN_DUPLICATE_LOCAL_VARS (1 << 1)
47 #define QP_WARN_UNKNOWN_WARNING (1 << 2)
48 #define QP_WARN_UNDECLARED_VAR (1 << 3)
49 #define QP_WARN_DUPLICATE_GLOBAL_VARS (1 << 4)
50 #define QP_WARN_UNREACHABLE_CODE (1 << 5)
51 #define QP_WARN_NONEXISTENT_METHOD_CALL (1 << 6)
52 #define QP_WARN_INVALID_OPERATION (1 << 7)
53 #define QP_WARN_CALL_WITH_TYPE_ERRORS (1 << 8)
54 #define QP_WARN_RETURN_VALUE_IGNORED (1 << 9)
55 #define QP_WARN_DEPRECATED (1 << 10)
56 #define QP_WARN_EXCESS_ARGS (1 << 11)
57 #define QP_WARN_DUPLICATE_HASH_KEY (1 << 12)
58 #define QP_WARN_UNREFERENCED_VARIABLE (1 << 13)
59 #define QP_WARN_DUPLICATE_BLOCK_VARS (1 << 14)
60 #define QP_WARN_MODULE_ONLY (1 << 15)
61 #define QP_WARN_BROKEN_LOGIC_PRECEDENCE (1 << 16)
62 #define QP_WARN_ALL -1
64 #define QP_WARN_MODULES (QP_WARN_UNREACHABLE_CODE|QP_WARN_NONEXISTENT_METHOD_CALL|QP_WARN_INVALID_OPERATION|QP_WARN_CALL_WITH_TYPE_ERRORS|QP_WARN_RETURN_VALUE_IGNORED|QP_WARN_DUPLICATE_HASH_KEY|QP_WARN_DUPLICATE_BLOCK_VARS|QP_WARN_BROKEN_LOGIC_PRECEDENCE)
66 #define QP_WARN_DEFAULT (QP_WARN_UNKNOWN_WARNING|QP_WARN_MODULES|QP_WARN_DEPRECATED)
68 enum BreakpointPolicy :
unsigned char {
75 DLLEXPORT
extern const char** qore_warnings;
78 DLLEXPORT
extern unsigned qore_num_warnings;
81 DLLEXPORT
int get_warning_code(
const char* str);
92 class FunctionCallNode;
93 class AbstractStatement;
97 class UserFunctionVariant;
98 class QoreParseTypeInfo;
100 class AbstractQoreZoneInfo;
101 class qore_program_private;
104 class AbstractQoreFunctionVariant;
106 class QoreExternalFunction;
107 class QoreExternalGlobalVar;
110 typedef std::list<QoreBreakpoint*> bkp_list_t;
112 hashdecl QoreBreakpointList_t :
public bkp_list_t {
113 DLLEXPORT QoreBreakpointList_t();
115 DLLEXPORT ~QoreBreakpointList_t();
127 friend class qore_program_private_base;
128 friend class qore_program_private;
129 friend class qore_debug_program_private;
130 friend hashdecl ThreadLocalProgramData;
133 qore_program_private* priv;
211 DLLEXPORT
void parseAndRun(FILE *fp,
const char* name);
223 DLLEXPORT
void parseAndRun(
const char* str,
const char* name);
260 DLLEXPORT
void parseAndRunClass(FILE *fp,
const char* name,
const char* classname);
273 DLLEXPORT
void parseAndRunClass(
const char* str,
const char* name,
const char* classname);
332 DLLEXPORT
void parse(
const char* str,
const char* lstr,
ExceptionSink* xsink,
ExceptionSink* warn_sink,
int warn_mask,
const char* source,
int offset);
588 DLLEXPORT
const AbstractQoreZoneInfo *
currentTZ()
const;
591 DLLEXPORT
void setTZ(
const AbstractQoreZoneInfo *n_TZ);
624 DLLEXPORT
void parseDefine(
const char* str,
const char* val);
627 DLLEXPORT
void parseCmdLineDefines(
const std::map<std::string, std::string> defmap,
ExceptionSink& xs,
ExceptionSink& ws,
int w);
635 DLLEXPORT
void parseCmdLineDefines(
ExceptionSink& xs,
ExceptionSink& ws,
int w,
const std::map<std::string, std::string>& defmap);
749 DLLEXPORT
const QoreExternalFunction*
findFunction(
const char* path)
const;
812 DLLLOCAL LocalVar *createLocalVar(
const char* name,
const QoreTypeInfo *typeInfo);
817 DLLLOCAL
void addFile(
char* f);
819 DLLLOCAL
void parseSetIncludePath(
const char* path);
820 DLLLOCAL
const char* parseGetIncludePath()
const;
827 DLLLOCAL
const LVList* getTopLevelLVList()
const;
835 DLLLOCAL
bool parseExceptionRaised()
const;
865 DLLEXPORT AbstractStatement*
findStatement(
const char* fileName,
int line)
const;
876 DLLEXPORT
unsigned long getStatementId(
const AbstractStatement* statement)
const;
969 DLLEXPORT
operator bool()
const;
976 void*
operator new(size_t) =
delete;
994 void*
operator new(size_t) =
delete;
1022 typedef std::list<AbstractStatement*> AbstractStatementList_t;
1023 typedef std::list<int> TidList_t;
1032 qore_program_private* pgm;
1033 AbstractStatementList_t statementList;
1034 typedef std::map<
int,
int> TidMap_t;
1038 static QoreBreakpointList_t breakpointList;
1039 static volatile unsigned breakpointIdCounter;
1040 unsigned breakpointId;
1042 DLLLOCAL
void unassignAllStatements();
1043 DLLLOCAL
bool isStatementAssigned(
const AbstractStatement *statement)
const;
1046 friend class qore_program_private;
1047 friend class AbstractStatement;
1133 DLLEXPORT
void setQoreObject(
QoreObject* n_qo);
1150 class ProgramThreadCountContextHelper* priv;
1165 class QoreExternalProgramCallContextHelperPriv* priv;
1168 #endif // _QORE_QOREPROGRAM_H
DLLEXPORT void disableParseOptions(int po, ExceptionSink *xsink)
turns off the parse options given in the passed mask and adds Qore-language exception information if ...
DLLEXPORT AbstractQoreProgramExternalData * removeExternalData(const char *owner)
removes a pointer to external data in the Program; does not dereference the data
DLLEXPORT void assignProgram(QoreProgram *new_pgm, ExceptionSink *xsink)
DLLEXPORT QoreProgram()
creates the object
DLLEXPORT unsigned long getStatementId(const AbstractStatement *statement) const
get the statement id
DLLEXPORT void setExternalData(const char *owner, AbstractQoreProgramExternalData *pud)
sets a pointer to external data in the Program
DLLEXPORT QoreProgram * programRefSelf() const
references "this" and returns a non-const pointer to itself
DLLEXPORT void registerQoreObject(QoreObject *o, ExceptionSink *xsink) const
register link to Qore script object
supports parsing and executing Qore-language code, reference counted, dynamically-allocated only
Definition: QoreProgram.h:126
DLLEXPORT void parseAndRun(FILE *fp, const char *name)
parses the given file and runs the file
DLLEXPORT int setGlobalVarValue(const char *name, QoreValue val, ExceptionSink *xsink)
sets the value of the given global variable
DLLEXPORT QoreListNode * runtimeFindCallVariants(const char *name, ExceptionSink *xsink) const
DLLEXPORT void parsePending(const char *code, const char *label, ExceptionSink *xsink, ExceptionSink *warn_sink=0, int warn_mask=QP_WARN_ALL)
parses code from the given string but does not commit changes to the QoreProgram
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
DLLEXPORT void getBreakpoints(QoreBreakpointList_t &bkptList)
DLLEXPORT QoreValue getLocalVariableVal(const char *var, bool &found) const
This is the hash or associative list container type in Qore, dynamically allocated only,...
Definition: QoreHashNode.h:50
DLLEXPORT void assignBreakpoint(QoreBreakpoint *bkpt, ExceptionSink *xsink)
DLLEXPORT QoreExternalProgramCallContextHelper(QoreProgram *pgm)
sets the call context to the given program
DLLEXPORT void depDeref()
dereferences a weak reference for the program object
DLLEXPORT QoreStringNode * getScriptDir() const
returns the script directory, if known (0 if not)
DLLEXPORT QoreProgramHelper(ExceptionSink &xs)
creates the QoreProgram object: DEPRECATED: use QoreProgramHelper(int64, ExceptionSink&) instead
DLLEXPORT void replaceParseOptions(int64 po, ExceptionSink *xsink)
replaces the parse options in the program with those given by the argument; adds Qore-language except...
const DLLEXPORT AbstractQoreFunctionVariant * runtimeFindCall(const char *name, const QoreListNode *params, ExceptionSink *xsink) const
DLLEXPORT QoreBreakpoint & operator=(const QoreBreakpoint &other)
DLLEXPORT void getThreadIds(TidList_t &tidList, ExceptionSink *xsink)
defines a Qore-language class
Definition: QoreClass.h:239
DLLEXPORT bool checkAllowDebugging(ExceptionSink *xsink)
check if program can provide debugging stuff
DLLEXPORT hashdecl_vec_t findAllHashDeclsRegex(const QoreString &pattern, int re_opts, ExceptionSink *xsink) const
returns a list of all typed hashes (hashdecls) that match the pattern
DLLEXPORT void addThreadId(int tid, ExceptionSink *xsink)
DLLEXPORT QoreExternalProgramContextHelper(ExceptionSink *xsink, QoreProgram *pgm)
try to attach to the given program, if not possible, then a Qore-language exception is thrown
DLLEXPORT void getStatements(AbstractStatementList_t &statList, ExceptionSink *xsink)
DLLEXPORT void parseSetParseOptions(int po)
adds the parse options given to the parse option mask; DEPRECATED: use parseSetParseOptions(int64) in...
const DLLEXPORT TypedHashDecl * findHashDecl(const char *path, const QoreNamespace *&pns) const
search for the given typed hash (hashdecl) in the program; can be a simple function name or a namespa...
DLLEXPORT AbstractStatement * resolveStatementId(unsigned long statementId) const
get the statement from statement id
DLLEXPORT void waitForTerminationAndDeref(ExceptionSink *xsink)
this call blocks until the program's last thread terminates, and then calls QoreProgram::deref()
DLLEXPORT QoreNamespace * getQoreNS() const
returns a pointer to the "Qore" namespace
DLLEXPORT void parseDisableParseOptions(int64 po)
disables the parse options given to the parse option mask
allows for the parse lock for the current program to be acquired by binary modules
Definition: QoreProgram.h:960
DLLEXPORT void setScriptPath(const char *path)
sets the script path
DLLEXPORT class_vec_t findAllClassesRegex(const QoreString &pattern, int re_opts, ExceptionSink *xsink) const
returns a list of all classes that match the pattern
DLLEXPORT void parseDefine(const char *str, QoreValue val)
defines a parse-time variable; call only at parse time (or before parsing)
DLLEXPORT ~CurrentProgramRuntimeExternalParseContextHelper()
releases the parse lock for the current program
DLLEXPORT RootQoreNamespace * getRootNS() const
returns a pointer to the root namespace
This is the list container type in Qore, dynamically allocated only, reference counted.
Definition: QoreListNode.h:52
DLLEXPORT QoreProgram * operator*()
returns the QoreProgram object being managed
DLLEXPORT bool existsFunction(const char *name)
returns true if the given function exists as a user function, false if not
DLLEXPORT ~QoreProgramHelper()
waits until all background threads in the Qore library have terminated and until the QoreProgram obje...
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
const DLLEXPORT QoreExternalFunction * findFunction(const char *path) const
search for the given function in the program; can be a simple function name or a namespace-prefixed p...
DLLEXPORT unsigned getProgramId() const
get the program id
DLLEXPORT AbstractStatement * findFunctionStatement(const char *functionName, const QoreListNode *params, ExceptionSink *xsink) const
Class implementing breakpoint for debugging.
Definition: QoreProgram.h:1030
const DLLEXPORT QoreClass * findClass(const char *path, ExceptionSink *xsink) const
search for the given class in the program; can be a simple class name or a namespace-prefixed path (e...
DLLEXPORT bool checkFeature(const char *f) const
returns true if the given feature is present in the program object
DLLEXPORT int issueModuleCmd(const char *module, const char *cmd, ExceptionSink *xsink)
issues a module command for the given module; the module is loaded into the current Program object if...
contains constants, classes, and subnamespaces in QoreProgram objects
Definition: QoreNamespace.h:65
DLLEXPORT int disableWarning(int code)
disables a warning by its code
DLLEXPORT QoreHashNode * getThreadData()
returns the thread-local data for the current thread and the Program object
static DLLEXPORT QoreProgram * resolveProgramId(unsigned programId)
get the program from program id
virtual DLLLOCAL ~QoreProgram()
the destructor is private in order to prohibit the object from being allocated on the stack
external wrapper class for constants
Definition: QoreReflection.h:200
DLLEXPORT gvar_vec_t findAllGlobalVarsRegex(const QoreString &pattern, int re_opts, ExceptionSink *xsink) const
returns a list of all global variables that match the pattern
Qore's string type supported by the QoreEncoding class.
Definition: QoreString.h:81
DLLEXPORT unsigned getBreakpointId() const
get the breakpoint id
DLLEXPORT int64 getParseOptions64() const
returns the parse options currently set for this program
DLLEXPORT QoreProgram * operator->()
returns the QoreProgram object being managed
DLLEXPORT void parseCommit(ExceptionSink *xsink, ExceptionSink *warn_sink=0, int warn_mask=QP_WARN_ALL)
commits pending changes to the program
const DLLEXPORT QoreExternalGlobalVar * findGlobalVar(const char *path, const QoreNamespace *&pns) const
search for the given global variable in the program; can be a simple function name or a namespace-pre...
DLLEXPORT QoreProgramContextHelper(QoreProgram *pgm)
sets the current Program context
DLLEXPORT void parse(FILE *fp, const char *name, ExceptionSink *xsink, ExceptionSink *warn_sink=0, int warn_mask=QP_WARN_ALL)
parses code from the file given and commits changes to the QoreProgram
DLLEXPORT QoreValue callFunction(const char *name, const QoreListNode *args, ExceptionSink *xsink)
calls a function from the function name and returns the return value
DLLEXPORT void assignStatement(AbstractStatement *statement, ExceptionSink *xsink)
virtual AbstractQoreProgramExternalData * copy(QoreProgram *pgm) const =0
for reference-counted classes, returns the same object with the reference count incremented
DLLEXPORT void depRef()
incremements the weak reference count for the program object
DLLEXPORT const_vec_t findAllNamespaceConstantsRegex(const QoreString &pattern, int re_opts, ExceptionSink *xsink) const
returns a list of all namespace constants that match the pattern
const DLLEXPORT QoreExternalConstant * findNamespaceConstant(const char *path, const QoreNamespace *&pns) const
search for the given namespace constant in the program; can be a simple function name or a namespace-...
typed hash declaration
Definition: TypedHashDecl.h:44
DLLEXPORT QoreStringNode * getScriptPath() const
returns the script path (directory and name), if known (0 if not)
DLLEXPORT QoreHashNode * getSourceLabels(ExceptionSink *xsink) const
get list of labels which appears in a statement
DLLEXPORT QoreStringNode * getScriptName() const
returns the script file name, if known (0 if not)
DLLEXPORT void unregisterQoreObject(QoreObject *o, ExceptionSink *xsink) const
unregister link to Qore script object
DLLEXPORT ns_vec_t findAllNamespacesRegex(const QoreString &pattern, int re_opts, ExceptionSink *xsink) const
returns a list of all namespaces that match the pattern
virtual DLLLOCAL bool checkBreak() const
check if program flow should be interrupted
DLLEXPORT QoreListNode * getUserFunctionList()
returns a list of all user functions in this program
DLLEXPORT void parseFileAndRunClass(const char *filename, const char *classname)
parses the given filename and runs the program by instantiating the class given
DLLEXPORT QoreHashNode * getSourceFileNames(ExceptionSink *xsink) const
get list of files which appears in a statement
DLLEXPORT void setThreadIds(TidList_t tidList, ExceptionSink *xsink)
const DLLEXPORT AbstractQoreZoneInfo * currentTZ()
returns the current local time zone, note that if 0 = UTC
the implementation of Qore's object data type, reference counted, dynamically-allocated only
Definition: QoreObject.h:61
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
DLLEXPORT QoreHashNode * getGlobalVars() const
retrieves a hash of global variables and their values
static DLLEXPORT QoreListNode * getAllQoreObjects(ExceptionSink *xsink)
list all programs as QoreObject list
const DLLLOCAL char * parseGetScriptDir() const
returns the script directory, if known (0 if not), does not grab the parse lock, only to be called wh...
DLLEXPORT void parseRollback()
rolls back changes to the program object that were added with QoreProgram::parsePending()
DLLEXPORT QoreListNode * getStatementIds(ExceptionSink *xsink)
DLLEXPORT int setWarningMask(int wm)
sets the warning mask
DLLEXPORT QoreValue getGlobalVariableValue(const char *var, bool &found) const
returns the value of the global variable given (do not include the "$" symbol), the caller owns the r...
allows the program call context to be set by external modules
Definition: QoreProgram.h:1156
DLLEXPORT void lockOptions()
locks parse options so they may not be changed
DLLEXPORT bool isThreadId(int tid, ExceptionSink *xsink)
provides a mutually-exclusive thread lock
Definition: QoreThreadLock.h:49
DLLEXPORT void removeThreadId(int tid, ExceptionSink *xsink)
DLLEXPORT void addFeature(const char *name)
manually add the feature to the program
DLLEXPORT void unassignStatement(AbstractStatement *statement, ExceptionSink *xsink)
DLLEXPORT void getStatementBreakpoints(const AbstractStatement *statement, QoreBreakpointList_t &bkptList)
DLLEXPORT void parseFileAndRun(const char *filename)
parses the given filename and runs the file
allows for external modules to set the current Program context explicitly
Definition: QoreProgram.h:982
DLLEXPORT CurrentProgramRuntimeExternalParseContextHelper()
acquires the parse lock; if already acquired by another thread, then this call blocks until the lock ...
static DLLEXPORT QoreBreakpoint * resolveBreakpointId(unsigned breakpointId)
get the breakpoint from breakpoint id
an unresolved call reference, only present temporarily in the parse tree
Definition: CallReferenceNode.h:68
BreakpointPolicy policy
Definition: QoreProgram.h:1057
DLLEXPORT int getParseOptions() const
returns the parse options currently set for this program; DEPRECATED; use getParseOptions64() instead
DLLEXPORT ~QoreExternalProgramCallContextHelper()
resets the call context to the original state
DLLEXPORT bool checkWarning(int code) const
returns true if the warning code is set
DLLEXPORT QoreNamespace * findNamespace(const QoreString &path)
search for the given namespace in the program; can be a simple namespace name or a namespace-prefixed...
DLLEXPORT QoreObject * findQoreObject() const
find Qore script object related to QoreProgram instance
virtual DLLLOCAL void deref()
decrements the reference count of the object without the possibility of throwing a Qore-language exce...
Definition: AbstractPrivateData.h:67
DLLEXPORT int enableWarning(int code)
enables a warning by its code
an abstract class for program-specific external data
Definition: QoreProgram.h:1006
DLLEXPORT QoreValue run(ExceptionSink *xsink)
runs the program (instantiates the program class if a program class has been set) and returns the ret...
DLLEXPORT void setExecClass(const char *ecn=0)
sets the name of the application class to be executed (instantiated) instead of top-level code
safely manages QoreProgram objects; note the the destructor will block until all background threads i...
Definition: QoreProgram.h:933
DLLEXPORT QoreValue getGlobalVariableVal(const char *var, bool &found) const
returns the value of the global variable given (do not include the "$" symbol), the caller owns the r...
DLLEXPORT void parseFile(const char *filename, ExceptionSink *xsink, ExceptionSink *warn_sink=0, int warn_mask=QP_WARN_ALL, bool only_first_except=false)
parses code from the file given and commits changes to the QoreProgram
DLLEXPORT QoreListNode * getFeatureList() const
returns a list of features in the program object
DLLEXPORT QoreListNode * getThreadList() const
returns a list of threads active in this Program object
DLLEXPORT void parseSetTimeZone(const char *zone)
sets the time zone during parsing
DLLEXPORT QoreProgram * getProgram()
returns the current QoreProgram
the base class for all data to be used as private data of Qore objects
Definition: AbstractPrivateData.h:44
DLLEXPORT void waitForTermination()
this call blocks until the program's last thread terminates
DLLEXPORT AbstractQoreProgramExternalData * getExternalData(const char *owner) const
retrieves the external data pointer
DLLEXPORT AbstractStatement * resolveStatementId(unsigned long statementId, ExceptionSink *xsink) const
DLLEXPORT void parseAndRunClass(FILE *fp, const char *name, const char *classname)
parses the given file and runs the code by instantiating the class given
the root namespace of a QoreProgram object
Definition: QoreNamespace.h:335
allows a program to be used and guarantees that it will stay valid until the destructor is run if suc...
Definition: QoreProgram.h:1141
DLLEXPORT func_vec_t findAllFunctionsRegex(const QoreString &pattern, int re_opts, ExceptionSink *xsink) const
returns a list of all functions that match the pattern
DLLEXPORT ~QoreProgramContextHelper()
restores the previous Program context
Qore's string value type, reference counted, dynamically-allocated only.
Definition: QoreStringNode.h:50
virtual void doDeref()=0
for non-reference counted classes, deletes the object immediately
static DLLEXPORT QoreObject * getQoreObject(QoreProgram *pgm)
get QoreObject of QoreProgram
DLLEXPORT ~QoreExternalProgramContextHelper()
destroys the object and releases the program to be destroyed if it was successfully acquired in the c...
DLLEXPORT void deleteAllBreakpoints()
DLLEXPORT QoreValue runTopLevel(ExceptionSink *xsink)
tuns the top level code and returns any return value
DLLEXPORT void setParseOptions(int po, ExceptionSink *xsink)
sets the parse options and adds Qore-language exception information if an error occurs
provides a simple POSIX-threads-based read-write lock
Definition: QoreRWLock.h:47
DLLEXPORT void runClass(const char *classname, ExceptionSink *xsink)
instantiates the class given and runs its constructor
DLLEXPORT int getWarningMask() const
returns the warning mask
DLLEXPORT void clearThreadIds(ExceptionSink *xsink)
DLLEXPORT AbstractStatement * findStatement(const char *fileName, int line) const
base class for call references, reference-counted, dynamically allocated only
Definition: CallReferenceNode.h:39