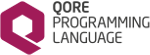 |
Qore Programming Language
0.9.16
|
34 #ifndef _QORE_QORESTRING_H
36 #define _QORE_QORESTRING_H
48 #define CE_HTML (1 << 0)
50 #define CE_XML (1 << 1)
52 #define CE_NONASCII (1 << 2)
54 #define CE_XHTML (CE_HTML | CE_XML)
56 #define CE_ALL (CE_XHTML | CE_NONASCII)
64 #define CD_HTML (1 << 0)
66 #define CD_XML (1 << 1)
68 #define CD_NUM_REF (1 << 2)
70 #define CD_XHTML (CD_HTML | CD_XML)
72 #define CD_ALL (CD_XHTML | CD_NUM_REF)
82 friend hashdecl qore_string_private;
157 DLLEXPORT
void set(
char* nbuf,
size_t nlen,
size_t nallocated,
const QoreEncoding* enc);
247 DLLEXPORT
void concatEscape(
const char* str,
char c,
char esc_char =
'\\');
353 DLLEXPORT
void concat(
const char* str);
356 DLLEXPORT
void concat(
const std::string& str);
362 DLLEXPORT
void concat(
const char c);
384 DLLEXPORT
int compare(
const char* str)
const;
394 DLLEXPORT
bool equal(
const char* str)
const;
444 DLLEXPORT
int sprintf(
const char* fmt, ...);
449 DLLEXPORT
int vsprintf(
const char* fmt, va_list args);
454 DLLEXPORT
void take(
char* str);
486 DLLEXPORT
void clear();
491 DLLEXPORT
void reset();
496 DLLEXPORT
void replaceAll(
const char* old_str,
const char* new_str);
610 DLLEXPORT
void tolwr();
615 DLLEXPORT
void toupr();
630 DLLEXPORT
const char*
c_str()
const;
635 DLLEXPORT
void allocate(
unsigned requested_size);
655 DLLEXPORT
void addch(
char c,
unsigned times);
699 DLLEXPORT
void trim(
const char* chars = 0);
724 DLLEXPORT
void trim(
char c);
780 DLLEXPORT
void prepend(
const char* str);
798 DLLEXPORT
bool operator==(
const std::string& other)
const;
801 DLLEXPORT
bool operator==(
const char* other)
const;
805 return !(*
this == other);
810 return !(*
this == other);
815 return !(*
this == other);
833 DLLEXPORT
bool empty()
const;
913 DLLLOCAL
QoreString(
struct qore_string_private* p);
917 hashdecl qore_string_private*
priv =
nullptr;
931 DLLLOCAL
void concat_reverse(
QoreString* targ)
const;
933 DLLLOCAL
int snprintf(
size_t size,
const char* fmt, ...);
934 DLLLOCAL
int vsnprintf(
size_t size,
const char* fmt, va_list args);
944 DLLEXPORT QoreStringMaker(
const char* fmt, ...);
949 DLLEXPORT QoreStringMaker(
const QoreEncoding* enc,
const char* fmt, ...);
952 DLLLOCAL QoreStringMaker(
const QoreStringMaker& str) =
delete;
953 DLLLOCAL QoreStringMaker&
operator=(
const QoreStringMaker&) =
delete;
993 DLLLOCAL
operator bool()
const {
return str !=
nullptr; }
1007 void*
operator new(size_t) =
delete;
1034 set_intern(&s, qe, xsink);
1044 set_intern(s, qe, xsink);
1069 set_intern(s, qe, xsink);
1070 return str !=
nullptr;
1096 DLLLOCAL
operator bool()
const {
return str != 0; }
1121 DLLLOCAL
void*
operator new(size_t) =
delete;
1124 DLLLOCAL
void discard_intern() {
1149 DLLEXPORT
size_t qore_get_unicode_character_width(
int ucs);
DLLEXPORT unsigned int getUnicodePointFromBytePos(qore_size_t offset, unsigned &len, ExceptionSink *xsink) const
return Unicode code point for the given byte offset
DLLEXPORT int64 toBigInt() const
returns the value of the string as an int64
DLLEXPORT char * giveBuffer()
returns the character buffer and leaves the QoreString empty, the caller owns the memory returned (mu...
DLLEXPORT bool equal(const QoreString &str) const
returns true if the strings are equal, false if not, if the character encodings are different,...
DLLEXPORT ~QoreString()
frees any memory allocated by the string
DLLEXPORT void concatHex(const char *buf, qore_size_t size)
concatenates hexidecimal digits corresponding to the binary data passed up to byte "len"
DLLEXPORT qore_offset_t index(const QoreString &needle, qore_offset_t pos, ExceptionSink *xsink) const
returns the character position of a substring within the string or -1 if not found
DLLEXPORT qore_offset_t find(char c, qore_offset_t pos=0) const
returns the byte position of a character (byte) within the string or -1 if not found
DLLEXPORT void reset()
reset string to zero length; memory is deallocated; string encoding is reset to QCS_DEFAULT
DLLEXPORT void clear()
reset string to zero length; memory is not deallocated; string encoding does not change
DLLEXPORT qore_offset_t brindex(const QoreString &needle, qore_offset_t pos) const
returns the byte position of a substring within the string searching in reverse from a given position...
DLLEXPORT qore_offset_t getByteOffset(qore_size_t i, ExceptionSink *xsink) const
returns the byte position of the given character position in the string or -1 if the string does not ...
DLLEXPORT void allocate(unsigned requested_size)
Ensure the internal buffer has at least expected size in bytes.
DLLEXPORT int trimLeading(ExceptionSink *xsink, const QoreString *chars=nullptr)
removes leading whitespace or other characters
DLLLOCAL QoreString * operator*()
returns the QoreString pointer being managed
Definition: QoreString.h:990
DLLEXPORT qore_size_t size() const
returns number of bytes in the string (not including the null pointer)
intptr_t qore_offset_t
used for offsets that could be negative
Definition: common.h:76
const DLLEXPORT QoreEncoding * QCS_DEFAULT
the default encoding for the Qore library
const DLLEXPORT QoreEncoding * getEncoding() const
returns the encoding for the string
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
DLLEXPORT void concatUTF8FromUnicode(unsigned code)
append a UTF-8 character sequence from a unicode code point, assumes the string is tagged with QCS_UT...
DLLEXPORT int concatDecodeUriRequest(const QoreString &url, ExceptionSink *xsink)
concatenates a URI-decoded version of the c-string passed
DLLLOCAL ~TempEncodingHelper()
deletes any temporary string being managed by the object
Definition: QoreString.h:1052
DLLEXPORT QoreString * reverse() const
return a Qorestring with the characters reversed
DLLEXPORT QoreString()
creates an empty string and assigns the default encoding QCS_DEFAULT
DLLEXPORT void trim_trailing(const char *chars=0)
remove trailing whitespace or other characters
DLLEXPORT char operator[](qore_offset_t pos) const
returns the byte (not character) at the given location; if the location is invalid,...
size_t qore_size_t
used for sizes (same range as a pointer)
Definition: common.h:73
DLLEXPORT void toupr()
converts the string to upper-case in place
DLLEXPORT bool operator==(const QoreString &other) const
returns true if the other string is equal to this string (encodings also must be equal)
DLLLOCAL QoreString * release()
releases the QoreString pointer being managed and sets the internal pointer to 0
Definition: QoreString.h:996
DLLEXPORT void concatEscape(const QoreString *str, char c, char esc_char, ExceptionSink *xsink)
concatenates a string and escapes character c with esc_char (converts encodings if necessary)
DLLEXPORT void concatBase64(const char *buf, qore_size_t size)
concatenates the base64-encoded version of the binary data passed
DLLEXPORT void trim_single_trailing(char c)
remove a single trailing character if present
DLLLOCAL char * giveBuffer()
returns a char pointer of the string, the caller owns the pointer returned (it must be manually freed...
Definition: QoreString.h:1102
DLLEXPORT qore_offset_t rfindAny(const char *str, qore_offset_t pos=-1) const
returns the last byte position of any of the given characters (bytes) within the string or -1 if not ...
DLLLOCAL int set(const QoreString *s, const QoreEncoding *qe, ExceptionSink *xsink)
discards any current state and sets and converts (if necessary) a new string to the desired encoding
Definition: QoreString.h:1066
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
DLLEXPORT bool equalPartialSoft(const QoreString &str, ExceptionSink *xsink) const
returns true if the beginning of the current string matches the argument string, false if not,...
DLLEXPORT QoreString * convertEncoding(const QoreEncoding *nccs, ExceptionSink *xsink) const
converts the encoding of the string to the specified encoding, returns 0 if an error occurs,...
DLLLOCAL void makeTemp()
ensures that the object is holding a temporary value
Definition: QoreString.h:1079
const DLLLOCAL QoreString * operator->()
returns the string being managed
Definition: QoreString.h:1087
DLLEXPORT bool equalSoft(const QoreString &str, ExceptionSink *xsink) const
returns true if the strings are equal, false if not, if the character encodings are different,...
DLLEXPORT unsigned int getUnicodePoint(qore_offset_t offset, ExceptionSink *xsink) const
return Unicode code point for the single character at the given character (not byte) offset in the st...
DLLEXPORT int sprintf(const char *fmt,...)
this will concatentate a formatted string to the existing string according to the format string and t...
DLLEXPORT void concatISO8601DateTime(const DateTime *d)
concatenates a DateTime value to a string in the format YYYYMMDDTHH:mm:SS <- where the "T" is a liter...
DLLEXPORT void concatAndHTMLEncode(const char *str)
concatenates HTML-encoded version of the c-string passed
DLLLOCAL bool operator!=(const QoreString &other) const
returns true if the other string is not equal to this string (encodings also must be equal)
Definition: QoreString.h:804
Qore's string type supported by the QoreEncoding class.
Definition: QoreString.h:81
const DLLEXPORT char * c_str() const
returns the string's buffer; this data should not be changed
class used to hold a possibly temporary QoreString pointer, stack only, cannot be dynamically allocat...
Definition: QoreString.h:966
DLLEXPORT int insert(const char *str, qore_size_t pos)
inserts a character string at a certain position in the string
DLLEXPORT void tolwr()
converts the string to lower-case in place
DLLEXPORT void prepend(const char *str)
prepends the string given to the string, assumes character encoding is the same as the string's
DLLEXPORT void splice(qore_offset_t offset, ExceptionSink *xsink)
removes characters from the string starting at position "offset"
DLLEXPORT void terminate(qore_size_t size)
terminates the string at byte position "size", the string is reallocated if necessary
DLLLOCAL bool is_temp() const
returns true if a temporary string is being managed
Definition: QoreString.h:1074
DLLEXPORT int concatEncodeUrl(ExceptionSink *xsink, const QoreString &url, bool encode_all=false)
concatenates a URL-encoded version of the c-string passed
DLLLOCAL QoreString * operator->()
returns the QoreString pointer being managed
Definition: QoreString.h:987
DLLEXPORT bool isDataAscii() const
returns true if the string is empty or has no characters with the high bit set (ie all characters < 1...
DLLEXPORT int trimTrailing(ExceptionSink *xsink, const QoreString *chars=nullptr)
removes trailing whitespace or other characters
DLLEXPORT void addch(char c, unsigned times)
append a character to the string a number of times
DLLEXPORT int concatUnicode(unsigned code, ExceptionSink *xsink)
append a character sequence from a unicode code point (returns 0 for OK, -1 for exception)
DLLEXPORT void replace(qore_size_t offset, qore_size_t len, const char *str)
replaces bytes with the string passed
const DLLEXPORT char * getBuffer() const
returns the string's buffer; this data should not be changed
DLLEXPORT QoreString & operator+=(const char *str)
concatenates the characters to the string; assumes the string to be concatenated is already in the ch...
DLLEXPORT void concat(const QoreString *str, ExceptionSink *xsink)
concatenates a string and converts encodings if necessary
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
use this class to manage strings where the character encoding must be specified and may be different ...
Definition: QoreString.h:1025
DLLEXPORT QoreString * copy() const
returns an exact copy of the string
DLLEXPORT QoreString * substr(qore_offset_t offset, ExceptionSink *xsink) const
returns a new string consisting of all the characters from the current string starting with character...
DLLEXPORT QoreString * extract(qore_offset_t offset, ExceptionSink *xsink)
removes characters from the string starting at position "offset" and returns a string of the characte...
DLLEXPORT int insertch(char c, qore_size_t pos, unsigned times)
insert a character at a certain position in the string a number of times
DLLEXPORT unsigned int getUnicodePointFromUTF8(qore_offset_t offset=0) const
return Unicode code point for character offset, string must be UTF-8
DLLEXPORT bool isDataPrintableAscii() const
returns true if the string is empty or only contains printable non-control ASCII characters (ie all c...
DLLEXPORT int compareSoft(const QoreString *str, ExceptionSink *xsink) const
compares the string with another string, performing character set encoding conversion if necessary
DLLEXPORT void concatAndHTMLDecode(const QoreString *str)
concatenates HTML-decoded version of the c-string passed
DLLEXPORT void trim_single_leading(char c)
remove a single leading character if present
DLLEXPORT qore_size_t strlen() const
returns number of bytes in the string (not including the null pointer)
DLLEXPORT qore_offset_t findAny(const char *str, qore_offset_t pos=0) const
returns the byte position of any of the given characters (bytes) within the string or -1 if not found
DLLEXPORT int vsprintf(const char *fmt, va_list args)
this will concatentate a formatted string to the existing string according to the format string and t...
DLLEXPORT bool equalPartialPath(const QoreString &str, ExceptionSink *xsink) const
returns true if the begining of the current string matches the argument string where either both stri...
Holds absolute and relative date/time values in Qore with precision to the microsecond.
Definition: DateTime.h:93
DLLEXPORT void removeBom()
remove any leading byte order marker (BOM) from UTF-16* strings
DLLEXPORT int concatDecode(ExceptionSink *xsink, const QoreString &str, unsigned code=CD_ALL)
concatenates a string and decodes HTML, XML, and numeric character references as per the supplied arg...
DLLEXPORT void take(char *str)
takes ownership of the character pointer passed and assigns it to the string (frees memory previously...
DLLLOCAL TempString()
populates the object with a new QoreString that this object will manage
Definition: QoreString.h:969
DLLEXPORT qore_size_t length() const
returns the number of characters (not bytes) in the string
DLLEXPORT int compare(const QoreString *str) const
compares two strings without converting encodings (if the encodings do not match then "this" is deeme...
DLLEXPORT QoreString & operator=(const QoreString &other)
assigns the value of one string to another
DLLEXPORT int concatEncodeUriRequest(ExceptionSink *xsink, const QoreString &url)
concatenates a URI-encoded version of the c-string passed
const DLLLOCAL QoreString * operator*()
returns the string being managed
Definition: QoreString.h:1090
DLLEXPORT int concatEncode(ExceptionSink *xsink, const QoreString &str, unsigned code=CE_XHTML)
concatenates a string and encodes it according to the encoding argument passed
DLLEXPORT BinaryNode * parseHex(ExceptionSink *xsink) const
parses the current string data as hexadecimal-encoded data and returns it as a BinaryNode pointer (ca...
DLLEXPORT void replaceChar(qore_size_t offset, char c)
replaces a byte with the byte passed
DLLLOCAL ~TempString()
deletes the QoreString pointer being managed
Definition: QoreString.h:982
DLLEXPORT QoreString * parseBase64ToString(ExceptionSink *xsink) const
parses the current string data as base64-encoded data and returns it as a QoreString pointer owned by...
DLLEXPORT qore_size_t capacity() const
returns number of bytes allocated for the string's buffer, capacity is always >= size
DLLEXPORT bool equalPartial(const QoreString &str) const
returns true if the beginning of the current string matches the argument string, false if not,...
DLLEXPORT bool empty() const
returns true if the string is empty, false if not
DLLEXPORT qore_offset_t bindex(const QoreString &needle, qore_offset_t pos) const
returns the byte position of a substring within the string or -1 if not found
DLLEXPORT BinaryNode * parseBase64(ExceptionSink *xsink) const
parses the current string data as base64-encoded data and returns it as a BinaryNode pointer (caller ...
defines string encoding functions in Qore
Definition: QoreEncoding.h:83
holds arbitrary binary data
Definition: BinaryNode.h:41
DLLLOCAL TempEncodingHelper()
creates an empty TempEncodingHelperObject that may be initialized with TempEncodingHelper::set() late...
Definition: QoreString.h:1048
DLLEXPORT void setEncoding(const QoreEncoding *new_encoding)
changes the tagged encoding to the given encoding; does not affect the actual string buffer,...
hashdecl qore_string_private * priv
the private implementation of QoreString
Definition: QoreString.h:917
DLLEXPORT void concatDecodeUrl(const char *url)
concatenates a URL-decoded version of the c-string passed
DLLEXPORT void trim_leading(const char *chars=0)
remove leading whitespace or other characters
DLLEXPORT void replaceAll(const char *old_str, const char *new_str)
replaces all occurences of the first string with the second string
DLLEXPORT size_t getCharWidth(ExceptionSink *xsink) const
returns the character width of the string
DLLEXPORT void set(const char *str, const QoreEncoding *new_qorecharset=QCS_DEFAULT)
copies the c-string passed and sets the value of the string and its encoding
DLLEXPORT void trim(const char *chars=0)
remove leading and trailing whitespace or other characters
DLLEXPORT qore_size_t chomp()
removes a single \n\r or \n from the end of the string and returns the number of characters removed
DLLEXPORT qore_offset_t rfind(char c, qore_offset_t pos=-1) const
returns the last byte position of a character (byte) within the string or -1 if not found
DLLEXPORT qore_offset_t rindex(const QoreString &needle, qore_offset_t pos, ExceptionSink *xsink) const
returns the character position of a substring searching in reverse from a given position or -1 if not...
DLLEXPORT void reserve(qore_size_t size)
ensures that at least the given size is available in the string; the string's contents are not affect...
DLLEXPORT void takeAndTerminate(char *str, qore_size_t size)
takes ownership of the character pointer passed and assigns it to the string (frees memory previously...