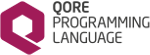 |
Qore Programming Language
0.9.16
|
32 #ifndef _QORE_INTERN_FUNCTIONREFERENCENODE_H
34 #define _QORE_INTERN_FUNCTIONREFERENCENODE_H
40 hashdecl CallReferenceNodeLocation {
41 const QoreProgramLocation* loc;
43 DLLLOCAL CallReferenceNodeLocation(
const QoreProgramLocation* loc) : loc(loc) {
49 DLLLOCAL AbstractCallReferenceNodeIntern(
const QoreProgramLocation* loc,
bool n_needs_eval) :
56 DLLLOCAL ResolvedCallReferenceNodeIntern(
const QoreProgramLocation* loc,
bool n_needs_eval =
false) :
61 class AbstractUnresolvedCallReferenceNode :
public AbstractCallReferenceNodeIntern {
63 DLLLOCAL AbstractUnresolvedCallReferenceNode(
const QoreProgramLocation* loc,
bool n_needs_eval) : AbstractCallReferenceNodeIntern(loc, n_needs_eval) {
76 DLLLOCAL
virtual void parseInit(
QoreValue& val, LocalVar* oflag,
int pflag,
int& lvids,
const QoreTypeInfo*& typeInfo);
84 DLLLOCAL
virtual void parseInit(
QoreValue& val, LocalVar* oflag,
int pflag,
int& lvids,
const QoreTypeInfo*& typeInfo);
120 const qore_class_private* class_ctx;
125 DLLLOCAL StaticMethodCallReferenceNode(
const QoreProgramLocation* loc,
const QoreMethod *n_method,
QoreProgram *n_pgm,
const qore_class_private* n_class_ctx);
127 DLLLOCAL ~StaticMethodCallReferenceNode() {
167 DLLLOCAL MethodCallReferenceNode(
const QoreProgramLocation* loc,
const QoreMethod* n_method,
QoreProgram* n_pgm);
186 return const_cast<QoreFunction*
>(uf);
190 const QoreFunction* uf;
226 DLLLOCAL
void deref() {
231 DLLLOCAL
virtual void parseInit(
QoreValue& val, LocalVar* oflag,
int pflag,
int& lvids,
const QoreTypeInfo*& typeInfo);
234 class ImportedFunctionEntry;
241 const qore_class_private* qc;
270 const qore_class_private* qc;
276 const QoreMethod* n_method,
const qore_class_private* ctx = runtime_get_class());
virtual DLLLOCAL QoreValue execValue(const QoreListNode *args, ExceptionSink *xsink) const
pure virtual function for executing the function reference
virtual DLLLOCAL void parseInit(QoreValue &val, LocalVar *oflag, int pflag, int &lvids, const QoreTypeInfo *&typeInfo)
for use by parse types to initialize them for execution during stage 1 parsing; not exported in the l...
supports parsing and executing Qore-language code, reference counted, dynamically-allocated only
Definition: QoreProgram.h:126
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
virtual DLLLOCAL bool is_equal_hard(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
virtual DLLLOCAL bool is_equal_hard(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
a call reference to a static user method
Definition: CallReferenceNode.h:135
virtual DLLLOCAL QoreProgram * getProgram() const
returns a pointer to the QoreProgram object associated with this reference (can be nullptr)
virtual DLLEXPORT bool derefImpl(ExceptionSink *xsink)
decrements the reference count
a run-time call reference to a method of a particular object
Definition: CallReferenceNode.h:237
virtual DLLLOCAL bool is_equal_hard(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
virtual DLLLOCAL bool derefImpl(ExceptionSink *xsink)
decrements the reference count
This is the list container type in Qore, dynamically allocated only, reference counted.
Definition: QoreListNode.h:52
an unresolved static method call reference, only present temporarily in the parse tree
Definition: CallReferenceNode.h:216
virtual DLLLOCAL QoreValue evalImpl(bool &needs_deref, ExceptionSink *xsink) const
this function should never be called for function references; this function should never be called di...
a call reference to a static user method
Definition: CallReferenceNode.h:88
virtual DLLLOCAL QoreValue execValue(const QoreListNode *args, ExceptionSink *xsink) const
pure virtual function for executing the function reference
virtual DLLLOCAL bool is_equal_soft(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
Definition: CallReferenceNode.h:151
virtual DLLLOCAL bool is_equal_hard(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
DLLEXPORT bool ROdereference() const
atomically decrements the reference count
a call reference to a user function
Definition: CallReferenceNode.h:199
a call reference to a user function from within the same QoreProgram object
Definition: CallReferenceNode.h:173
virtual DLLLOCAL bool is_equal_soft(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
Definition: CallReferenceNode.h:179
virtual DLLLOCAL QoreValue execValue(const QoreListNode *args, ExceptionSink *xsink) const
pure virtual function for executing the function reference
virtual DLLLOCAL bool is_equal_hard(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
DLLEXPORT void depRef()
incremements the weak reference count for the program object
virtual DLLLOCAL void parseInit(QoreValue &val, LocalVar *oflag, int pflag, int &lvids, const QoreTypeInfo *&typeInfo)
for use by parse types to initialize them for execution during stage 1 parsing; not exported in the l...
virtual DLLLOCAL QoreProgram * getProgram() const
returns a pointer to the QoreProgram object associated with this reference (can be nullptr)
virtual DLLLOCAL QoreFunction * getFunction()
Returns the internal function object, if any; can return nullptr.
Definition: CallReferenceNode.h:185
the implementation of Qore's object data type, reference counted, dynamically-allocated only
Definition: QoreObject.h:61
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
virtual DLLLOCAL QoreValue execValue(const QoreListNode *args, ExceptionSink *xsink) const
pure virtual function for executing the function reference
virtual DLLLOCAL QoreValue execValue(const QoreListNode *args, ExceptionSink *xsink) const
pure virtual function for executing the function reference
virtual DLLLOCAL QoreFunction * getFunction()
Returns the internal function object, if any; can return nullptr.
Definition: CallReferenceNode.h:258
virtual DLLLOCAL QoreValue evalImpl(bool &needs_deref, ExceptionSink *xsink) const
this function should never be called for function references; this function should never be called di...
virtual DLLLOCAL QoreValue evalImpl(bool &needs_deref, ExceptionSink *xsink) const
this function should never be called for function references; this function should never be called di...
an unresolved call reference, only present temporarily in the parse tree
Definition: CallReferenceNode.h:68
virtual DLLLOCAL bool is_equal_soft(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
Definition: CallReferenceNode.h:282
a run-time call reference to a method of a particular object where the method's class
Definition: CallReferenceNode.h:266
virtual DLLLOCAL bool is_equal_soft(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
Definition: CallReferenceNode.h:252
DLLEXPORT void deref(ExceptionSink *xsink)
decrements the reference count and calls derefImpl() if there_can_be_only_one is false,...
a method in a QoreClass
Definition: QoreClass.h:125
virtual DLLLOCAL QoreFunction * getFunction()
Returns the internal function object, if any; can return nullptr.
base class for resolved call references
Definition: CallReferenceNode.h:105
virtual DLLLOCAL bool is_equal_soft(const AbstractQoreNode *v, ExceptionSink *xsink) const
returns true if the other node is the same value
Definition: CallReferenceNode.h:106
The base class for all value and parse types in Qore expression trees.
Definition: AbstractQoreNode.h:54
virtual DLLLOCAL QoreValue execValue(const QoreListNode *args, ExceptionSink *xsink) const
pure virtual function for executing the function reference
virtual DLLLOCAL QoreFunction * getFunction()
Returns the internal function object, if any; can return nullptr.
base class for call references, reference-counted, dynamically allocated only
Definition: CallReferenceNode.h:39