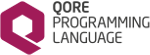 |
Qore Programming Language
0.9.16
|
Go to the documentation of this file.
32 #ifndef _QORE_NODE_TYPES_H
34 #define _QORE_NODE_TYPES_H
90 #define QORE_NUM_TYPES 45
93 #define NUM_SIMPLE_TYPES 8
96 #define NUM_VALUE_TYPES 12
const qore_type_t NT_IMPLICIT_ARG
type value for QoreImplicitArgumentNode (private class)
Definition: node_types.h:72
const qore_type_t NT_BACKQUOTE
type value for BackquoteNode
Definition: node_types.h:81
const qore_type_t NT_NUMBER
type value for QoreNumberNode
Definition: node_types.h:53
const qore_type_t NT_STATIC_METHOD_CALL
type value for StaticMethodCallNode (private class)
Definition: node_types.h:74
const qore_type_t NT_METHOD_CALL
type value for MethodCallNode (private class)
Definition: node_types.h:73
const qore_type_t NT_OBJECT
type value for QoreObject
Definition: node_types.h:52
const qore_type_t NT_PROGRAM_FUNC_CALL
type value for ProgramFunctionCallNode (private class)
Definition: node_types.h:79
const qore_type_t NT_CONTEXT_ROW
type value for ContextRowNode
Definition: node_types.h:65
const qore_type_t NT_NOTHING
type value for QoreNothingNode
Definition: node_types.h:42
const qore_type_t NT_BOOLEAN
type value for bools (QoreValue only)
Definition: node_types.h:47
const qore_type_t NT_SELF_VARREF
type value for SelfVarrefNode
Definition: node_types.h:60
const qore_type_t NT_SELF_CALL
type value for SelfFunctionCallNode (private class)
Definition: node_types.h:75
const qore_type_t NT_COMPLEXCONTEXTREF
type value for ComplexContextrefNode
Definition: node_types.h:55
const qore_type_t NT_OBJMETHREF
type value for AbstractParseObjectMethodReferenceNode
Definition: node_types.h:67
const qore_type_t NT_FIND
type value for FindNode
Definition: node_types.h:58
const qore_type_t NT_DATE
type value for DateTimeNode
Definition: node_types.h:46
const qore_type_t NT_CLOSURE
type value for QoreClosureParseNode (private class)
Definition: node_types.h:70
const qore_type_t NT_FUNCREF
type value for AbstractCallReferenceNode
Definition: node_types.h:68
const qore_type_t NT_CLASSREF
type value for ClassRefNode
Definition: node_types.h:66
const qore_type_t NT_CONSTANT
type value for ScopedRefNode (private class)
Definition: node_types.h:62
const qore_type_t NT_FLOAT
type value for floating-point values (QoreValue only)
Definition: node_types.h:44
const qore_type_t NT_FUNCREFCALL
type value for CallReferenceCallNode
Definition: node_types.h:69
const qore_type_t NT_PARSE_NEW_COMPLEX_TYPE
type value for ParseNewComplexTypeNode
Definition: node_types.h:85
const qore_type_t NT_PARSEREFERENCE
type value for ParseReferenceNode (private class)
Definition: node_types.h:80
const qore_type_t NT_FUNCTION_CALL
type value for FunctionCallNode
Definition: node_types.h:59
const qore_type_t NT_INT
type value for integers (QoreValue only)
Definition: node_types.h:43
const qore_type_t NT_PARSE_HASH
type value for QoreParseHashNode
Definition: node_types.h:83
const qore_type_t NT_STRING
type value for QoreStringNode
Definition: node_types.h:45
const qore_type_t NT_NEW_HASHDECL
type value for NewHashDeclNode
Definition: node_types.h:86
const qore_type_t NT_REFERENCE
type value for ReferenceNode
Definition: node_types.h:64
const qore_type_t NT_RUNTIME_CLOSURE
type value for ResolvedCallReferenceNode (QoreClosureNode, QoreObjectClosureNode)
Definition: node_types.h:71
const qore_type_t NT_RTCONSTREF
type value for RuntimeConstantRefNode
Definition: node_types.h:82
const qore_type_t NT_CONTEXTREF
type value for ContextrefNode
Definition: node_types.h:54
const qore_type_t NT_PARSE_LIST
type value for QoreParseListNode
Definition: node_types.h:84
const qore_type_t NT_BAREWORD
type value for BarewordNode
Definition: node_types.h:63
const qore_type_t NT_NULL
type value for QoreNullNode
Definition: node_types.h:48
const qore_type_t NT_IMPLICIT_ELEMENT
type value for QoreImplicitElementNode (private clas)
Definition: node_types.h:77
const qore_type_t NT_WEAKREF
type value for WeakReferenceNode
Definition: node_types.h:87
const qore_type_t NT_OPERATOR
type value for QoreOperatorNode (private class)
Definition: node_types.h:76
const qore_type_t NT_LIST
type value for QoreListNode
Definition: node_types.h:50
const qore_type_t NT_VARREF
type value for VarRefNode
Definition: node_types.h:56
const qore_type_t NT_HASH
type value for QoreHashNode
Definition: node_types.h:51
const qore_type_t NT_BINARY
type value for BinaryNode
Definition: node_types.h:49
int16_t qore_type_t
used to identify unique Qore data and parse types (descendents of AbstractQoreNode)
Definition: common.h:70
const qore_type_t NT_TREE
type value for QoreTreeNode
Definition: node_types.h:57
const qore_type_t NT_CLASS_VARREF
type value for StaticClassVarRefNode (private class)
Definition: node_types.h:78
const qore_type_t NT_SCOPE_REF
type value for ScopedObjectCallNode
Definition: node_types.h:61