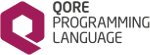 |
Qore Programming Language
0.9.16
|
Go to the documentation of this file.
31 #ifndef INCLUDE_QORE_INTERN_ICONVHELPER_H_
32 #define INCLUDE_QORE_INTERN_ICONVHELPER_H_
43 #ifdef NEED_ICONV_TRANSLIT
45 to_code.concat(
"//TRANSLIT");
46 c = iconv_open(to_code.getBuffer(), from->
getCode());
50 if (c == (iconv_t) -1) {
51 if (errno == EINVAL) {
52 xsink->
raiseException(
"ENCODING-CONVERSION-ERROR",
"cannot convert from \"%s\" to \"%s\"",
55 reportUnknownError(xsink);
60 DLLLOCAL ~IconvHelper() {
61 if (c != (iconv_t) -1) {
66 DLLLOCAL
size_t iconv(
char **inbuf,
size_t *inavail,
char **outbuf,
size_t *outavail) {
67 return iconv_adapter(::iconv, c, inbuf, inavail, outbuf, outavail);
70 void reportIllegalSequence(
size_t offset,
ExceptionSink *xsink) {
72 "illegal character sequence at byte offset " QLLD
" found in input type \"%s\" (while converting to \"%s\")",
77 xsink->
raiseErrnoException(
"ENCODING-CONVERSION-ERROR", errno,
"unknown error converting from \"%s\" to \"%s\"",
84 static size_t iconv_adapter(
size_t (*iconv_f)(iconv_t, T,
size_t *,
char **,
size_t *), iconv_t handle,
85 char **inbuf,
size_t *inavail,
char **outbuf,
size_t *outavail) {
86 return (*iconv_f) (handle,
const_cast<T
>(inbuf), inavail, outbuf, outavail);
95 #endif // INCLUDE_QORE_INTERN_ICONVHELPER_H_
DLLEXPORT AbstractQoreNode * raiseErrnoException(const char *err, int en, const char *fmt,...)
appends a Qore-language exception to the list and appends the result of strerror(errno) to the descri...
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
Qore's string type supported by the QoreEncoding class.
Definition: QoreString.h:81
DLLEXPORT AbstractQoreNode * raiseException(const char *err, const char *fmt,...)
appends a Qore-language exception to the list
const DLLEXPORT char * getCode() const
returns the string code (ex: "UTF-8") for the encoding
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
defines string encoding functions in Qore
Definition: QoreEncoding.h:83