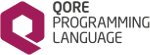 |
Qore Programming Language
0.9.16
|
32 #ifndef _QORE_QC_TREEMAP_H
33 #define _QORE_QC_TREEMAP_H
44 static inline bool isPathEnd(
char c) {
45 return c ==
'/' || c ==
'?';
48 static inline size_t getFirstPathSegmentLength(
const std::string& path) {
49 size_t prefixLen = path.find_first_of(
"/?");
50 return prefixLen == std::string::npos ? path.length() : prefixLen;
53 static inline bool isPrefix(
const std::string& prefix,
const std::string& str) {
54 return str.length() >= prefix.length() && !str.compare(0, prefix.length(), prefix);
57 static inline bool isPathPrefix(
const std::string& prefix,
const std::string& path) {
58 return isPrefix(prefix, path) && (path.length() == prefix.length() || isPathEnd(path[prefix.length()]));
63 DLLLOCAL TreeMapData() {
68 for (Map::iterator i = data.begin(), e = data.end(); i != e; ++i) {
69 i->second.discard(xsink);
80 Map::mapped_type &refToMap = data[keyStr->c_str()];
81 refToMap.discard(xsink);
95 std::string path(keyStr->c_str());
97 Map::const_iterator b = data.begin();
98 Map::const_iterator it = data.upper_bound(path);
100 size_t prefixLen = getFirstPathSegmentLength(path);
101 while (it != b && !(--it)->first.compare(0, prefixLen, path, 0, prefixLen)) {
102 if (isPathPrefix(it->first, path)) {
104 size_t l = it->first.length();
105 if (isPathEnd(path[l])) {
112 if (*xsink ||
ref.assign(path.release()))
115 return it->second.refSelf();
136 for (Map::const_iterator i = data.begin(), e = data.end(); i != e; ++i)
137 h->setKeyValue(i->first.c_str(), i->second.refSelf(),
nullptr);
149 Map::iterator i = data.find(keyStr->c_str());
159 typedef std::map<std::string, QoreValue> Map;
const DLLEXPORT QoreEncoding * QCS_DEFAULT
the default encoding for the Qore library
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
This is the hash or associative list container type in Qore, dynamically allocated only,...
Definition: QoreHashNode.h:50
defines a Qore-language class
Definition: QoreClass.h:239
DLLLOCAL void ref()
increments the reference count of the object
Definition: AbstractPrivateData.h:53
provides a safe and exception-safe way to hold read locks in Qore, only to be used on the stack,...
Definition: QoreRWLock.h:105
contains constants, classes, and subnamespaces in QoreProgram objects
Definition: QoreNamespace.h:65
DLLEXPORT bool ROdereference() const
atomically decrements the reference count
helper class to manage variable references passed to functions and class methods, stack only,...
Definition: QoreTypeSafeReferenceHelper.h:57
DLLEXPORT QoreValue refSelf() const
references the contained value if type == QV_Node, returns itself
DLLEXPORT QoreNothingNode Nothing
the global and unique NOTHING object in Qore
DLLEXPORT QoreStringNode * substr(qore_offset_t offset, ExceptionSink *xsink) const
returns a new string consisting of all the characters from the current string starting with character...
parse type: reference to a lvalue expression
Definition: ReferenceNode.h:45
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
use this class to manage strings where the character encoding must be specified and may be different ...
Definition: QoreString.h:1025
unsigned qore_classid_t
used for the unique class ID for QoreClass objects
Definition: common.h:79
provides a safe and exception-safe way to hold write locks in Qore, only to be used on the stack,...
Definition: QoreRWLock.h:144
virtual DLLLOCAL void deref()
decrements the reference count of the object without the possibility of throwing a Qore-language exce...
Definition: AbstractPrivateData.h:67
the base class for all data to be used as private data of Qore objects
Definition: AbstractPrivateData.h:44
Qore's string value type, reference counted, dynamically-allocated only.
Definition: QoreStringNode.h:50
provides a simple POSIX-threads-based read-write lock
Definition: QoreRWLock.h:47