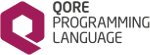 |
Qore Programming Language
0.9.16
|
34 #ifndef _QORE_QORE_SOCKET_OBJECT_H
36 #define _QORE_QORE_SOCKET_OBJECT_H
38 #include <qore/QoreSocket.h>
39 #include <qore/AbstractPrivateData.h>
40 #include <qore/QoreThreadLock.h>
49 friend class my_socket_priv;
50 friend hashdecl qore_httpclient_priv;
57 DLLLOCAL
virtual ~QoreSocketObject();
60 DLLEXPORT QoreSocketObject();
63 DLLEXPORT
virtual void deref();
65 DLLEXPORT
int connect(
const char* name,
int timeout_ms,
ExceptionSink* xsink = NULL);
66 DLLEXPORT
int connectINET(
const char* host,
int port,
int timeout_ms,
ExceptionSink* xsink = NULL);
67 DLLEXPORT
int connectINET2(
const char* host,
const char* service,
int family,
int sock_type,
int protocol,
int timeout_ms = -1,
ExceptionSink* xsink = NULL);
68 DLLEXPORT
int connectUNIX(
const char* p,
int socktype,
int protocol,
ExceptionSink* xsink = NULL);
69 DLLEXPORT
int connectSSL(
const char* name,
int timeout_ms,
ExceptionSink* xsink);
70 DLLEXPORT
int connectINETSSL(
const char* host,
int port,
int timeout_ms,
ExceptionSink* xsink);
71 DLLEXPORT
int connectINET2SSL(
const char* host,
const char* service,
int family,
int sock_type,
int protocol,
int timeout_ms = -1,
ExceptionSink* xsink = NULL);
72 DLLEXPORT
int connectUNIXSSL(
const char* p,
int socktype,
int protocol,
ExceptionSink* xsink);
74 DLLEXPORT
int bind(
const char* name,
bool reuseaddr =
false);
76 DLLEXPORT
int bind(
int port,
bool reuseaddr =
false);
78 DLLEXPORT
int bind(
const char* iface,
int port,
bool reuseaddr =
false);
80 DLLEXPORT
int bindUNIX(
const char* name,
int socktype,
int protocol,
ExceptionSink* xsink);
81 DLLEXPORT
int bindINET(
const char* name,
const char* service,
bool reuseaddr,
int family,
int socktype,
int protocol,
ExceptionSink* xsink);
84 DLLEXPORT
int getPort();
87 DLLEXPORT QoreSocketObject *accept(
int timeout_ms,
ExceptionSink* xsink);
88 DLLEXPORT QoreSocketObject *acceptSSL(
int timeout_ms,
ExceptionSink* xsink);
90 DLLEXPORT
int listen(
int backlog);
92 DLLEXPORT
int send(
const char* buf,
int size);
93 DLLEXPORT
int send(
const char* buf,
int size,
int timeout_ms,
ExceptionSink* xsink);
103 DLLEXPORT
int send(
int fd,
int size = -1);
105 DLLEXPORT
int sendi1(
char b,
int timeout_ms,
ExceptionSink* xsink);
106 DLLEXPORT
int sendi2(
short b,
int timeout_ms,
ExceptionSink* xsink);
107 DLLEXPORT
int sendi4(
int b,
int timeout_ms,
ExceptionSink* xsink);
109 DLLEXPORT
int sendi2LSB(
short b,
int timeout_ms,
ExceptionSink* xsink);
110 DLLEXPORT
int sendi4LSB(
int b,
int timeout_ms,
ExceptionSink* xsink);
124 DLLEXPORT
int recv(
int fd,
int size,
int timeout);
141 const char* http_version,
const QoreHashNode* headers,
const void* ptr,
int size,
int source,
int timeout_ms);
145 const char *path,
const char *http_version,
const QoreHashNode *headers,
150 const char* http_version,
const QoreHashNode* headers,
const void* data,
size_t size,
int source,
164 int source,
int timeout_ms,
bool* aborted =
nullptr);
167 DLLEXPORT
void sendHTTPChunkedBodyFromInputStream(
InputStream *is,
size_t max_chunked_size,
const int timeout_ms,
187 DLLEXPORT
int setSendTimeout(
int ms);
188 DLLEXPORT
int setRecvTimeout(
int ms);
189 DLLEXPORT
int getSendTimeout();
190 DLLEXPORT
int getRecvTimeout();
191 DLLEXPORT
int close();
192 DLLEXPORT
int shutdown();
194 DLLEXPORT
const char* getSSLCipherName();
195 DLLEXPORT
const char* getSSLCipherVersion();
196 DLLEXPORT
bool isSecure();
197 DLLEXPORT
long verifyPeerCertificate();
198 DLLEXPORT
int getSocket();
201 DLLEXPORT
bool isDataAvailable(
ExceptionSink* xsink,
int timeout = 0);
202 DLLEXPORT
bool isWriteFinished(
ExceptionSink* xsink,
int timeout = 0);
203 DLLEXPORT
bool isOpen()
const;
208 DLLEXPORT
int setNoDelay(
int nodelay);
209 DLLEXPORT
int getNoDelay();
211 DLLEXPORT
void setEventQueue(Queue* cbq,
ExceptionSink* xsink);
218 DLLEXPORT
void upgradeClientToSSL(
int timeout_ms,
ExceptionSink* xsink);
219 DLLEXPORT
void upgradeServerToSSL(
int timeout_ms,
ExceptionSink* xsink);
224 DLLEXPORT
void clearStats();
225 DLLEXPORT
bool pendingHttpChunkedBody()
const;
226 DLLEXPORT
void setSslVerifyMode(
int mode);
227 DLLEXPORT
int getSslVerifyMode()
const;
228 DLLEXPORT
void acceptAllCertificates(
bool accept_all =
true);
229 DLLEXPORT
bool getAcceptAllCertificates()
const;
230 DLLEXPORT
bool captureRemoteCertificates(
bool set);
231 DLLEXPORT
QoreObject* getRemoteCertificate()
const;
232 DLLEXPORT
int64 getConnectionId()
const;
235 #endif // _QORE_QORE_SOCKET_OBJECT_H
Interface for private data of output streams.
Definition: OutputStream.h:44
intptr_t qore_offset_t
used for offsets that could be negative
Definition: common.h:76
The main value class in Qore, designed to be passed by value.
Definition: QoreValue.h:262
This is the hash or associative list container type in Qore, dynamically allocated only,...
Definition: QoreHashNode.h:50
provides access to sockets using Qore data structures
Definition: QoreSocket.h:126
long long int64
64bit integer type, cannot use int64_t here since it breaks the API on some 64-bit systems due to equ...
Definition: common.h:260
represents an X509 certificate, reference-counted, dynamically-allocated only
Definition: QoreSSLCertificate.h:42
a helper class for getting socket origination information
Definition: QoreSocket.h:73
the implementation of Qore's object data type, reference counted, dynamically-allocated only
Definition: QoreObject.h:61
container for holding Qore-language exception information and also for registering a "thread_exit" ca...
Definition: ExceptionSink.h:48
virtual DLLLOCAL void deref()
decrements the reference count of the object without the possibility of throwing a Qore-language exce...
Definition: AbstractPrivateData.h:67
provides access to a private key data structure for SSL connections
Definition: QoreSSLPrivateKey.h:40
base class for resolved call references
Definition: CallReferenceNode.h:105
the base class for all data to be used as private data of Qore objects
Definition: AbstractPrivateData.h:44
defines string encoding functions in Qore
Definition: QoreEncoding.h:83
holds arbitrary binary data
Definition: BinaryNode.h:41
Qore's string value type, reference counted, dynamically-allocated only.
Definition: QoreStringNode.h:50
The base class for all value and parse types in Qore expression trees.
Definition: AbstractQoreNode.h:54